Design Patterns
They are called "design patterns" because they offer standardized solutions to common problems in software design. The term "pattern" in this context draws an analogy to patterns used in architecture and other fields of engineering and design.
Gang of four​
The Gang of Four (GoF) design patterns are a fundamental part of software engineering and are widely used in object-oriented software development.
Authors​
- Ralph Johnson
- Richard Helm
- Erich Gamma
- John Vlissides
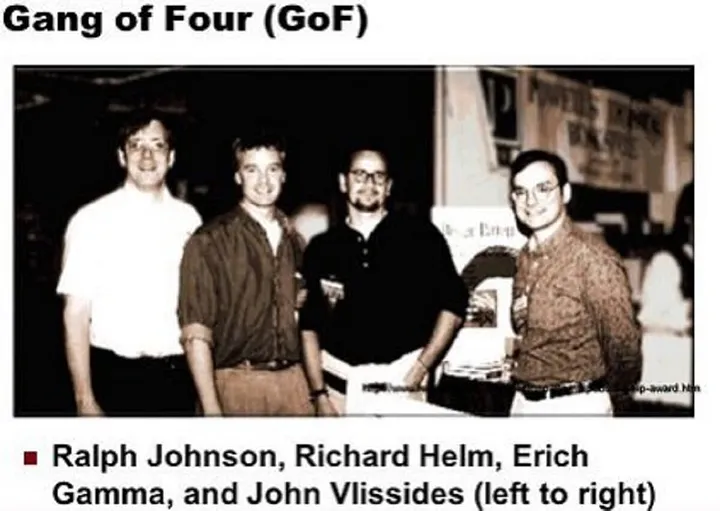
Categorization​
Creational​
Purpose: The purpose of these patterns is to abstract the instantiation process, making a system independent of how its objects are created, composed, and represented. This is particularly useful in scenarios where the system needs to be dynamic about the creation of objects.
Focus: Creational patterns in software design are focused on handling object creation mechanisms, optimizing the way objects are created in terms of flexibility and reusability.
Structural​
Purpose: Structural patterns are primarily concerned with how objects and classes are composed to form larger structures. They simplify the structure by identifying the relationships.
Focus: These patterns focus on simplifying the design by identifying a simple way to realize relationships between entities.
Behavioral​
Purpose: Behavioral patterns are concerned with algorithms and the assignment of responsibilities between objects. They focus on communication between objects.
Focus: These patterns are concerned with how objects interact and distribute responsibility.
Tips​
- When using structural patterns in React, think about how components are composed. For instance, the Composite pattern can be seen in how React components are nested.
- For behavioral patterns, consider how state and props are managed across components, resembling patterns like Observer (React's lifting state up) or Strategy (passing different algorithms as props).
- Utilize TypeScript for strong typing benefits, which can help in more effectively implementing these patterns by ensuring correct types are used across different structural or behavioral implementations.
- Always consider the maintainability and readability of your code when applying patterns, as the primary goal is to simplify and clarify your design, not to complicate it.
Creational Patterns​
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Defines an interface for creating an object, but lets subclasses alter the type of objects that will be created.
- Abstract Factory: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
- Prototype: Creates new objects by copying an existing object, known as the prototype.
Structural Patterns​
- Adapter: Allows incompatible interfaces to work together. It involves a wrapper that converts one interface to another.
- Bridge: Separates an object’s abstraction from its implementation, so the two can vary independently.
- Composite: Allows you to compose objects into tree structures to represent part-whole hierarchies.
- Decorator: Adds new functionalities to an object dynamically.
- Facade: Provides a simplified interface to a complex subsystem.
- Flyweight: Reduces the cost of creating and manipulating a large number of similar objects.
- Proxy: Provides a surrogate or placeholder for another object to control access to it.
Behavioral Patterns​
- Chain of Responsibility: Passes a request along a chain of handlers. Upon receiving a request, each handler decides either to process the request or to pass it to the next handler in the chain.
- Command: Encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations.
- Interpreter: Defines a grammatical representation for a language and provides an interpreter to deal with this grammar.
- Iterator: Provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation.
- Mediator: Defines an object that encapsulates how a set of objects interact.
- Memento: Allows an object to save and restore its internal state without revealing its implementation.
- Observer: Defines a dependency between objects so that when one object changes state, all its dependents are notified and updated automatically.
- State: Allows an object to alter its behavior when its internal state changes. The object will appear to change its class.
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable. Strategy lets the algorithm vary independently from clients that use it.
- Template Method: Defines the skeleton of an algorithm in an operation, deferring some steps to subclasses.
- Visitor: Lets you define a new operation without changing the classes of the elements on which it operates.
Tips for Fullstack Developers​
- Choose the Right Pattern: Understand the problem you are solving and choose a design pattern that fits. Not every problem requires a design pattern.
- Code Reusability: Design patterns can help in writing reusable and maintainable code. Try to recognize common scenarios where a pattern can be applied.
- Avoid Overcomplication: Sometimes, simpler code is better than a sophisticated design pattern. Don't use patterns just for the sake of it.
- Learn and Experiment: Continuously learn about new patterns and practices. Experiment with them in your projects to understand their pros and cons in different scenarios.
- Combine with Best Practices: Use design patterns in combination with other programming best practices and principles like SOLID and DRY.
Other Famous Patterns​
Each of these patterns serves a specific purpose in the design and development of software and is particularly useful in various scenarios in web development. By understanding and correctly applying these patterns, developers can create more efficient, maintainable, and scalable web applications.
Architectural Patterns​
These patterns provide a high-level structure for software applications.
-
MVC (Model-View-Controller): Organizes an application into three interconnected components to separate internal representations of information from how information is presented and accepted.
-
MVVM (Model-View-ViewModel): Similar to MVC but introduces a ViewModel for decoupling business logic and view logic.
-
Microservices Architecture: Involves developing a single application as a suite of small, independently deployable services.
-
Event-Driven Architecture (EDA): Centers around the production, detection, and reaction to events or changes in state.
Design Patterns​
These patterns provide solutions to common problems in software design at a lower level than architectural patterns.
-
Repository Pattern: Abstracts data access and manipulation in an application, promoting a more clear separation of concerns.
-
Service Layer Pattern: Defines an application's business logic layer, offering a way to organize complex business logic.
-
Active Record Pattern: An approach to accessing data in a database where an object contains both data and behavior.
-
CQRS (Command Query Responsibility Segregation): Separates read and write operations for more scalable and maintainable code architectures.
-
Page Object Pattern: Used in test automation, representing the screens of a web app as a series of objects within test scripts.
-
DTO (Data Transfer Object) Pattern: Used to transfer data between processes, reducing the number of method calls, especially in network environments.
Test Automation Pattern​
This category is specific to patterns used in automating the testing of software.
- Page Object Pattern (also listed under Design Patterns): Particularly useful in automating user interface tests for web applications.