Facade
The Facade Pattern is a structural design pattern that provides a simplified interface to a complex subsystem. Think of it as a "front-facing" interface masking more complex underlying or structural code.
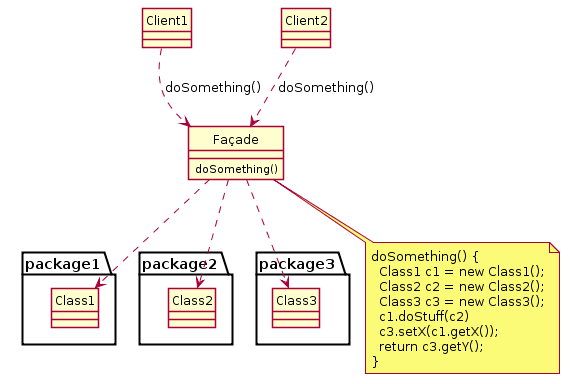
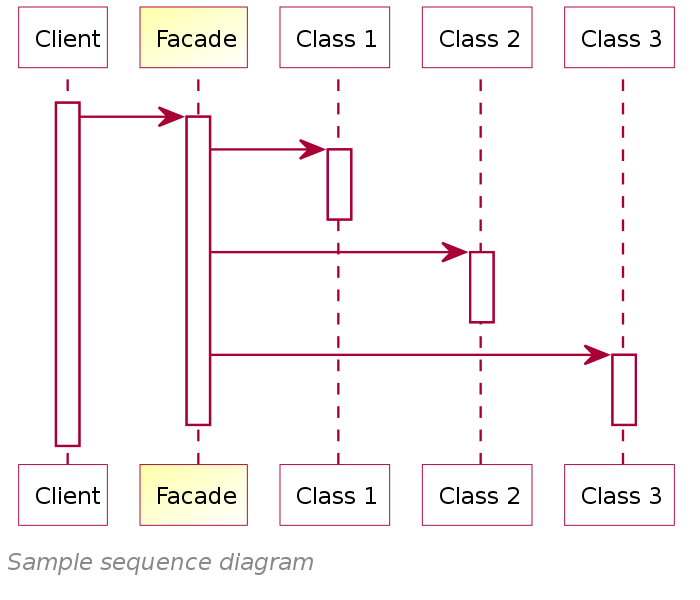
Facade patter in the Wikipedia
Why Use the Facade Pattern?​
- Simplicity: It simplifies the interface for clients. Instead of dealing with a complex system, the client interacts with a single, straightforward facade.
- Decoupling: It decouples a client implementation from the complex subsystem. Changes in the subsystem won't directly impact the client.
- Readability and Maintainability: It makes the code more readable and maintainable. With all the complexities hidden behind a facade, it's easier to understand and work with the system.
Real-World Example​
Imagine you're building a web application with complex logic for processing user data, generating reports, and sending notifications. Instead of having your web interface interact directly with all these different components, you create a UserFacade
class. This class provides simple methods like processUserData()
that internally handle all the complex interactions and processing.
How It Works in Code​
Here's a very basic example in TypeScript:
// Complex subsystem classes
class UserProcessor {
process(user: User) {
// complex logic...
}
}
class ReportGenerator {
generate(user: User) {
// complex report generation...
}
}
class NotificationSender {
send(user: User) {
// send notifications...
}
}
// Facade
class UserFacade {
private processor = new UserProcessor();
private reportGenerator = new ReportGenerator();
private notifier = new NotificationSender();
handleUser(user: User) {
this.processor.process(user);
this.reportGenerator.generate(user);
this.notifier.send(user);
}
}
// Client code
const userFacade = new UserFacade();
userFacade.handleUser(newUser);
In this example, UserFacade
simplifies the client's interaction with the underlying subsystems.
When to Use It​
- When you have a complex system and want to provide a simple interface to it.
- When there are many dependencies between clients and the implementation classes of an abstraction.
Caveats​
Be cautious not to create a "god object" facade that does too much, as it can become a bottleneck and a point of failure in your architecture.
It's not about eliminating complexity but abstracting it.
So, the Facade pattern is about creating a simple interface over a complex set of functionalities, making it easier for other parts of the system or the clients to interact with these functionalities. It's all about making things more manageable and approachable.
In FP​
Implementing the Facade pattern in a functional programming context is quite interesting because functional programming (FP) emphasizes stateless operations and the use of functions over objects. In FP, a facade can be viewed as a collection of functions that abstract away the complexities of a subsystem.
Concept in Functional Programming​
In FP, the Facade pattern doesn't revolve around a single class or object but around a module or a set of functions that serve as the simplified interface. This module encapsulates the complexities and offers a more straightforward, functional interface to the rest of the application.
Example: Functional Facade in TypeScript​
Let's adapt the previous example into a more functional style using TypeScript:
// Complex subsystem functions
const processUser = (user: User) => {
// complex logic...
};
const generateReport = (user: User) => {
// complex report generation...
};
const sendNotification = (user: User) => {
// send notifications...
};
// Facade as a set of functions
const handleUser = (user: User) => {
processUser(user);
generateReport(user);
sendNotification(user);
};
// Using the facade
handleUser(newUser);
In this functional approach:
- Subsystems are Represented as Functions: Each part of the subsystem is a function (
processUser
,generateReport
,sendNotification
). - Facade is a Function Too: The facade itself is a function (
handleUser
) that composes these subsystem functions to provide a simplified interface. - Statelessness: The functions are stateless. They take input and return output without side effects (in an ideal functional world).
Advantages in FP​
- Composability: Functions can be easily composed to create new functionalities.
- Reusability: Individual functions can be reused across different parts of the application.
- Testability: Stateless functions are easier to test due to predictable outputs for given inputs.
When to Use​
- When working in a functional programming environment and needing to simplify interactions with a complex set of functions.
- When you want to maintain the purity and composability of your functional code while dealing with complex logic.
Conclusion​
In functional programming, the Facade pattern is more about grouping and composing functions to create a simplified interface. It maintains the principles of FP like statelessness and function composition, making the complex parts of the system more manageable and accessible.