Bridge
The Bridge pattern is a structural design pattern used in software engineering. It aims to separate an abstraction from its implementation so that the two can vary independently. This promotes better code organization, scalability, and flexibility. The Bridge pattern is particularly useful in situations where you need to avoid a permanent binding between an abstraction and its implementation, which can be the case when the implementation must be selected or switched at runtime.
Components of the Bridge Pattern​
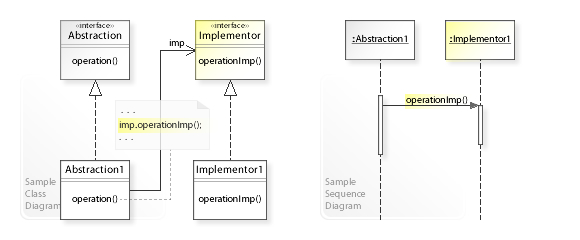
-
Abstraction: This is a high-level control layer for some entity. This layer is not supposed to do the actual work on its own. It delegates the work to the implementation layer.
-
Refined Abstraction: This is an extension of the abstraction layer. It overrides some of the operations to provide more specialized behavior.
-
Implementor: This defines the interface for the implementation classes. This interface doesn't have to correspond exactly to Abstraction's interface; it can be very different. Abstraction provides higher-level control, while Implementor provides the nuts and bolts of the platform-specific code.
-
Concrete Implementor: These are classes that implement the Implementor interface and define specific implementations.
How it Works​
- The "abstraction" class contains a reference to the "implementor" class.
- Abstraction delegates the work to the implementor.
- There can be many implementations of the implementor interface, and the abstraction's job is to use these implementations.
Example in TypeScript​
Consider a simple example of a remote control (as the abstraction) and a TV (as the implementor). The remote control can control different kinds of TVs.
// Implementor
interface Device {
isEnabled(): boolean;
enable(): void;
disable(): void;
getVolume(): number;
setVolume(percent: number): void;
// other operations like getChannel, setChannel
}
// Concrete Implementors
class TV implements Device {
// implementation for TV
}
class Radio implements Device {
// implementation for Radio
}
// Abstraction
class RemoteControl {
protected device: Device;
constructor(device: Device) {
this.device = device;
}
togglePower() {
if (this.device.isEnabled()) {
this.device.disable();
} else {
this.device.enable();
}
}
// other functions like volumeUp, volumeDown
}
// Refined Abstraction
class AdvancedRemoteControl extends RemoteControl {
// additional features
}
// Client code
let tv = new TV();
let remote = new RemoteControl(tv);
remote.togglePower();
let radio = new Radio();
let advancedRemote = new AdvancedRemoteControl(radio);
advancedRemote.togglePower();
Example in React​
In a React application, you might use the Bridge pattern when you have a component that needs to work with different data sources or services. The component (abstraction) would interact with a data source (implementor) through a common interface, allowing you to switch data sources without changing the component.
Benefits of Bridge Pattern​
-
Separation of Concerns: It separates the interface (abstraction) from its implementation.
-
Extensibility: Both the abstractions and implementors can be extended independently.
-
Runtime Binding: You can swap out implementations at runtime.
-
Platform Independence: Great for dealing with platform-specific components without affecting the client code.
When to Use​
- When you want to avoid a permanent binding between an abstraction and its implementation.
- When both the abstractions and their implementations can vary independently.
- In scenarios where implementation details should be hidden from the client.
The Bridge pattern encourages better organization and flexibility, making it a valuable pattern for full-stack development, particularly when working with various platforms and technologies.
FP version​
Creating a Functional Programming (FP) version of the Bridge pattern is a bit unconventional, as the Bridge pattern is inherently object-oriented, involving concepts like abstraction and implementation inheritance. However, we can still apply FP principles to achieve similar goals of decoupling and flexibility.
In FP, we focus more on functions and less on objects. We can represent abstractions and implementations using functions and higher-order functions instead of classes and interfaces.
Let's re-imagine the remote control and device example from an FP perspective:
TypeScript Example​
- Device Implementations as Functions: Instead of classes, we use functions to represent different devices.
interface Device {
isEnabled: () => boolean;
enable: () => void;
disable: () => void;
setVolume: (percent: number) => void;
// other device-specific functionalities
}
const TV = (): Device => ({
isEnabled: () => {/* ... */},
enable: () => {/* ... */},
disable: () => {/* ... */},
setVolume: (percent: number) => {/* ... */}
});
const Radio = (): Device => ({
isEnabled: () => {/* ... */},
enable: () => {/* ... */},
disable: () => {/* ... */},
setVolume: (percent: number) => {/* ... */}
});
- Abstraction as Higher-Order Functions: The remote control abstraction can be a higher-order function that takes a device and returns functions to control it.
const RemoteControl = (device: Device) => ({
togglePower: () => {
if (device.isEnabled()) {
device.disable();
} else {
device.enable();
}
},
volumeUp: () => {
let currentVolume = device.getVolume();
device.setVolume(currentVolume + 10);
},
// other control functions
});
- Usage: You can create specific devices and control them with the remote control functions.
const myTV = TV();
const myRemote = RemoteControl(myTV);
myRemote.togglePower();
// other operations
Key Takeaways​
-
Function Composition: This example leverages function composition, a core concept in FP, to achieve the same level of flexibility and decoupling as the Bridge pattern.
-
Higher-Order Functions: These are used to encapsulate the 'abstraction' part of the Bridge pattern, allowing for dynamic behavior based on the provided 'implementation' (device in this case).
-
Immutability and Statelessness: The FP approach encourages immutability and stateless functions, which are beneficial for predictable and maintainable code.
While this approach doesn't follow the traditional Bridge pattern, it aligns with the FP paradigm and achieves similar objectives of decoupling and flexibility. It's a demonstration of how OO patterns can be reinterpreted in a functional context, particularly relevant for a full-stack developer working with JavaScript/TypeScript and frameworks like React.