Flyweight
The Flyweight pattern is a structural design pattern used primarily to reduce the number of objects created, to decrease memory footprint and increase performance. This pattern is particularly useful when a program requires a large number of similar objects with few states that can be shared.
Concept​
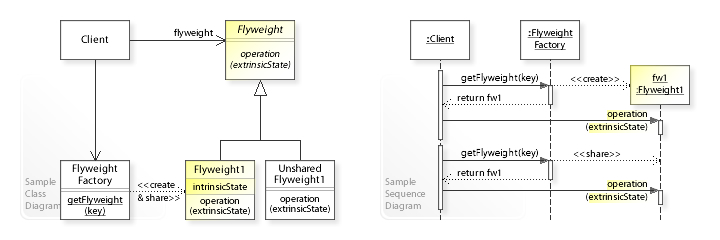
Flyweight pattern in the Wikipedia
- Intrinsic State: This is the state that is shared across many objects. It's stored in the flyweight object. This state is not unique to each object and is often immutable.
- Extrinsic State: This is the state that varies between objects and is not shared. Clients store this state and pass it to the flyweight when its behavior is invoked.
- Flyweight Factory: This is responsible for creating and managing flyweight objects. It ensures that flyweights are shared correctly. When the client requests a flyweight, the factory either returns an existing instance or creates a new one if it doesn't exist.
Use Cases​
The Flyweight pattern is particularly useful in scenarios where you're dealing with large numbers of objects that have some shared state. Examples include:
- Character objects in a word processor (where each character might have a font and size - the intrinsic state, but position and color - the extrinsic state).
- Trees in a forest simulation (where each tree might share species, texture, and color but have different positions and sizes).
Implementation in TypeScript​
class Flyweight {
private intrinsicState: any;
constructor(intrinsicState: any) {
this.intrinsicState = intrinsicState;
}
public operation(extrinsicState: any): void {
// Implementation of some action with intrinsic and extrinsic state
}
}
class FlyweightFactory {
private flyweights: {[key: string]: Flyweight} = {};
public getFlyweight(key: string): Flyweight {
if (!(key in this.flyweights)) {
this.flyweights[key] = new Flyweight(key);
}
return this.flyweights[key];
}
}
// Usage
const factory = new FlyweightFactory();
const flyweight1 = factory.getFlyweight("sharedState1");
const flyweight2 = factory.getFlyweight("sharedState1");
// Both flyweight1 and flyweight2 share the same intrinsic state
Common usage scenarios​
The Flyweight pattern is particularly useful in scenarios where a program must handle a large number of objects with many shared properties, allowing for efficient use of memory. Here are some common use scenarios:
-
Graphical Applications: In graphics software or games, where numerous objects are rendered, the Flyweight pattern can be used to reduce the memory footprint. For example, in a game, trees, bullets, or characters might share common properties like textures or models.
-
Text Formatting: Word processors or text editing software often use the Flyweight pattern. Characters in a document can share the font style, size, and color, which are intrinsic states, while the position of each character on a page is an extrinsic state.
-
User Interface Elements: In UI frameworks, the Flyweight pattern can be used to manage state and behavior of UI components that are repetitive but share common properties, such as buttons, icons, or text fields.
-
Database-Driven Applications: When dealing with large datasets, such as in database systems, flyweights can be used to represent shared data in a memory-efficient manner, reducing the load on the database.
-
Networking: In networked applications, such as web servers, the Flyweight pattern can be used to handle a large number of lightweight client sessions with shared settings or configurations.
-
Object Pooling: The Flyweight pattern is often used in conjunction with object pools, which manage the instantiation and lifecycle of a large number of objects of the same class.
Tips for Fullstack Developers​
- In a fullstack environment, consider using the Flyweight pattern to optimize memory usage on both the client and server sides.
- In a React application, you might apply this pattern for components that are reused frequently with similar properties, reducing the overall number of component instances.
- Be cautious about overusing this pattern, especially in scenarios where object uniqueness is more important than memory savings. The added complexity might not always justify the performance gains.
- When working with state management in frontend frameworks, the Flyweight pattern can help in efficiently managing shared state across multiple components.
- When implementing the Flyweight pattern in a web application, consider how you might use it to optimize performance, especially in the front-end (like React) where rendering large numbers of components can be expensive.
- In React, for instance, you might use this pattern to reuse component instances or share common data across components efficiently.
- Remember that while this pattern can improve performance by reducing memory usage, it can also add complexity. Use it only when the performance benefit is significant.