Abstract Factory
The Abstract Factory Pattern is a creational design pattern that provides an interface for creating families of related or dependent objects without specifying their concrete classes. This pattern is particularly useful in scenarios where a system needs to be independent of how its products are created, composed, and represented. It promotes consistency among products, as it ensures that related products can be used together.
Here's a breakdown of the key components in the Abstract Factory pattern:
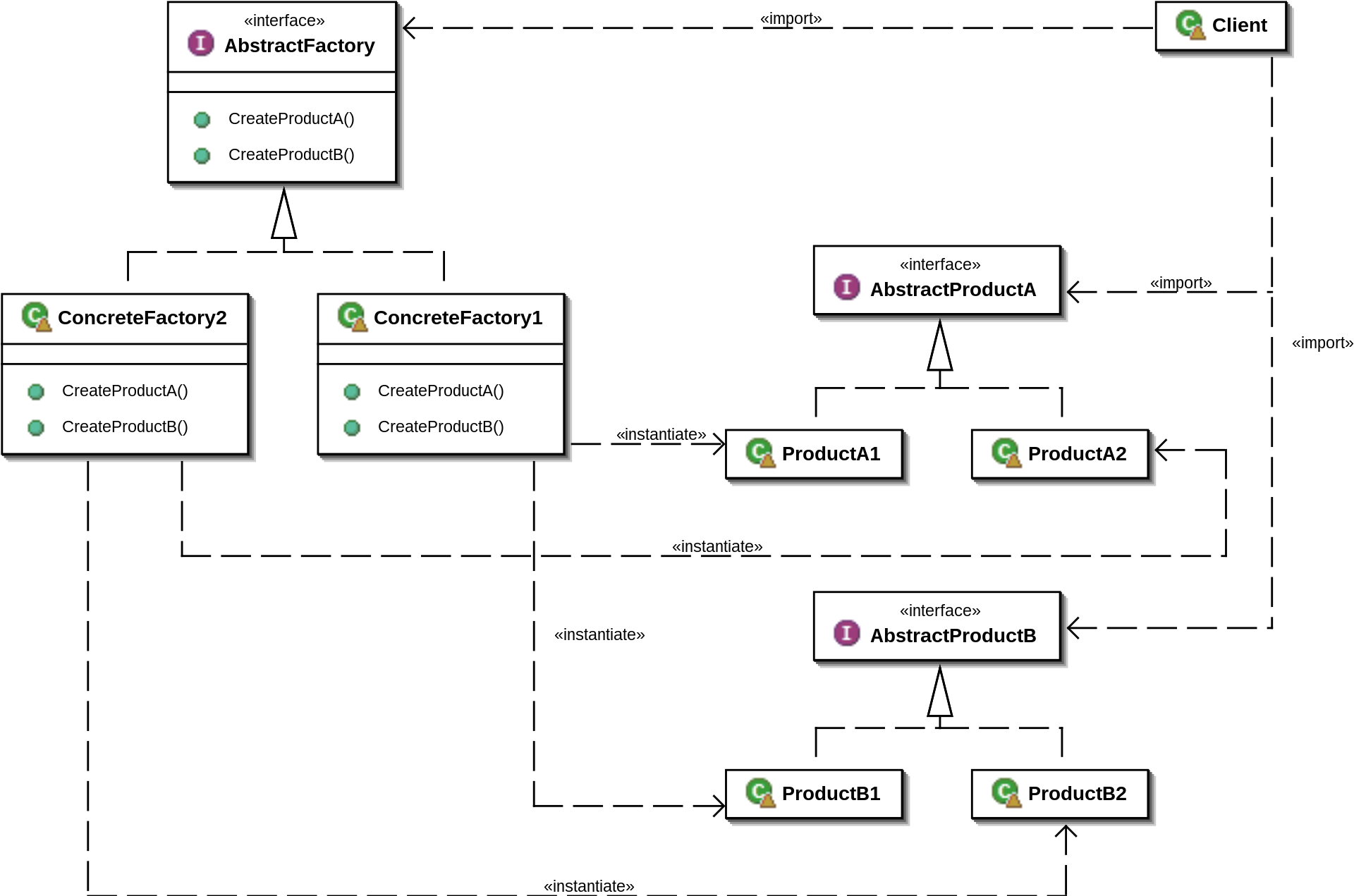
-
Abstract Factory: An interface with methods for creating abstract products.
-
Concrete Factory: Implements the operations declared in the Abstract Factory to create concrete products.
-
Abstract Product: Declares an interface for a type of product object.
-
Concrete Product: Implements the Abstract Product interface; objects of this type are created by the corresponding Concrete Factory.
-
Client: Uses only interfaces declared by the Abstract Factory and Abstract Product classes.
In a programming context, especially in TypeScript which you are familiar with, this pattern is useful for:
- Ensuring that a set of related products (or objects) is consistently created and used together.
- Hiding the details of object creation from the client, allowing for easy interchangeability of different families of objects.
Example in TypeScript​
Let's consider an example where we have two types of UI elements: Buttons and Checkboxes. These UI elements can have different styles, like Light and Dark themes.
// Abstract Products
interface Button {
paint(): void;
}
interface Checkbox {
paint(): void;
}
// Concrete Products
class LightButton implements Button {
paint() {
console.log('Rendering a light button');
}
}
class DarkButton implements Button {
paint() {
console.log('Rendering a dark button');
}
}
class LightCheckbox implements Checkbox {
paint() {
console.log('Rendering a light checkbox');
}
}
class DarkCheckbox implements Checkbox {
paint() {
console.log('Rendering a dark checkbox');
}
}
// Abstract Factory
interface GUIFactory {
createButton(): Button;
createCheckbox(): Checkbox;
}
// Concrete Factories
class LightThemeFactory implements GUIFactory {
createButton(): Button {
return new LightButton();
}
createCheckbox(): Checkbox {
return new LightCheckbox();
}
}
class DarkThemeFactory implements GUIFactory {
createButton(): Button {
return new DarkButton();
}
createCheckbox(): Checkbox {
return new DarkCheckbox();
}
}
// Client code
class Application {
private button: Button;
private checkbox: Checkbox;
constructor(factory: GUIFactory) {
this.button = factory.createButton();
this.checkbox = factory.createCheckbox();
}
paint() {
this.button.paint();
this.checkbox.paint();
}
}
// Using the factory
const darkThemeFactory = new DarkThemeFactory();
const app = new Application(darkThemeFactory);
app.paint();
In this example, the Application
class is not concerned with the specific types of buttons and checkboxes it uses. It works with the abstract Button
and Checkbox
interfaces, and objects of these types are instantiated by the factory passed to the application. Changing the theme of the application is as simple as using a different factory (LightThemeFactory
or DarkThemeFactory
), demonstrating the flexibility of the Abstract Factory pattern.
Tips for Fullstack Developers​
- When implementing design patterns in frontend development, especially with React, consider how these patterns can help in managing the complexity of your components and their interactions.
- The Abstract Factory pattern can be particularly useful in a micro-frontend architecture or when dealing with multiple themes or styles in your application.
- Always balance the use of design patterns with readability and maintainability of your code. Overuse of patterns can lead to unnecessarily complex code.
Compared to Factory Method​
The Factory Method and Abstract Factory patterns are both creational design patterns used in object-oriented programming, but they serve different purposes and are used in different scenarios. Understanding the differences between them can help you choose the right pattern for a particular situation, especially in your work as a fullstack developer.
Key Differences​
- Number of Products: Factory Method is about creating a single product, whereas Abstract Factory is about creating families of related or dependent products.
- Method of Creation: Factory Method uses inheritance and relies on a subclass to handle the desired object instantiation. Abstract Factory uses composition to delegate responsibility to different factory instances.
- Flexibility: Abstract Factory is more flexible as it can create multiple kinds of objects. Factory Method is simpler and is only focused on creating one type of object.
Tips for Fullstack Developers​
- Use the Factory Method when you have many objects that can be categorized into a single group and you only need one of them at a time.
- The Abstract Factory is more suitable when dealing with multiple related objects that need to work together.
- In a React context, Factory Method could be used for creating components dynamically, while Abstract Factory might be more useful when dealing with themes or a set of related components like a widget toolkit.
- Remember, while design patterns are powerful, they should be used judiciously. Overuse can lead to overly complex and hard-to-maintain code.