Factory Method
The Factory Method is a creational design pattern in object-oriented programming. It's used to create objects without specifying the exact class of the object that will be created. This pattern is particularly useful when the specific type of the object to be created is determined at runtime.
Here's a basic overview of the Factory Method pattern:
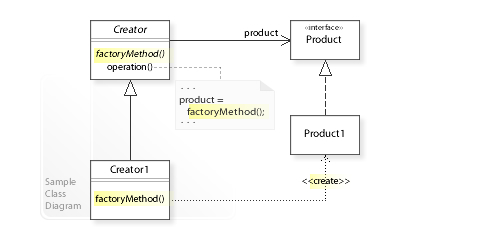
Factory Method pattern in the Wikipedia
-
Purpose: The main purpose is to define an interface for creating an object but let the subclasses decide which class to instantiate. This lets the coding to an interface rather than a specific class.
-
Implementation: The pattern involves a creator class with a method for creating objects. This method can be abstract (requiring subclasses to implement the object creation) or it can have a default implementation. The subclasses can then override this method to change the type of objects that will be created.
-
Benefits:
- Flexibility: The Factory Method pattern provides flexibility in the code as it allows the system to be independent of how its objects are created, composed, and represented.
- Scalability: It's easy to introduce new types of products without modifying existing client code.
- Encapsulation: Clients are decoupled from the specifics of the concrete products being created.
-
Use Cases: It is widely used when a class cannot anticipate the class of objects it must create, or when a class wants its subclasses to specify the objects it creates.
Here's a simple example in TypeScript:
interface Product {
operation(): string;
}
class ConcreteProduct1 implements Product {
operation(): string {
return 'Result of ConcreteProduct1';
}
}
class ConcreteProduct2 implements Product {
operation(): string {
return 'Result of ConcreteProduct2';
}
}
abstract class Creator {
public abstract factoryMethod(): Product;
public someOperation(): string {
const product = this.factoryMethod();
return `Creator: The same creator's code worked with ${product.operation()}`;
}
}
class ConcreteCreator1 extends Creator {
public factoryMethod(): Product {
return new ConcreteProduct1();
}
}
class ConcreteCreator2 extends Creator {
public factoryMethod(): Product {
return new ConcreteProduct2();
}
}
function clientCode(creator: Creator) {
console.log(creator.someOperation());
}
clientCode(new ConcreteCreator1());
clientCode(new ConcreteCreator2());
In this example, Creator
is an abstract class with the factoryMethod
. ConcreteCreator1
and ConcreteCreator2
are subclasses that implement this method to create different types of products (ConcreteProduct1
and ConcreteProduct2
). The clientCode
function shows how the factory method can be used to create objects without specifying the exact class.
Tip for React Developers​
In the context of React, you might not use the Factory Method pattern in the traditional sense, but the concept can be applied. For instance, when you have a component that needs to render different child components based on props or state, you can use a factory-like method to determine which component to render. This keeps your component code clean and makes it easier to add new types of child components in the future.
Dependency Inversion Principle (DIP)​
The Factory Method pattern is often used in conjunction with the Dependency Inversion Principle (DIP), one of the five SOLID principles of object-oriented design. The DIP states that:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
In the context of the Factory Method pattern:
-
Abstraction in Creation: The Factory Method pattern allows high-level modules (e.g., the client code or a service layer) to remain independent of the concrete classes of objects they need to create. The factory acts as an abstraction layer that encapsulates the object creation process.
-
Inversion of Control: With the Factory Method, the control over which object to create is inverted from the high-level module to the factory class. Instead of the high-level module instantiating concrete classes (which would create a direct dependency), it delegates this responsibility to the factory, which is designed to work with abstract classes or interfaces.
-
Flexibility and Decoupling: By using factories, your high-level components become decoupled from the specific classes they operate on. This means changes in the details of object creation (like introducing a new subclass) don't require changes in the high-level modules that use these objects. This adheres to the principle of "details depend on abstractions".
Example in TypeScript Context​
Suppose you're developing a web application with React and TypeScript. You could design a factory for creating various service objects that interact with different parts of your backend API. The high-level components in your application would interact with an abstract service interface, and the factory would determine the concrete implementation to instantiate, based on the context (such as user preferences, configuration, etc.).
This approach aligns with the Dependency Inversion Principle because your high-level modules depend on abstract services, not concrete implementations, and the details of creating these services are encapsulated within the factory.
Tip for Fullstack Development​
In a full-stack environment, especially when working with frameworks like React, incorporating design patterns like Factory Method and principles like DIP can lead to more maintainable and scalable codebases. It helps in managing dependencies and makes your code less susceptible to changes in business logic or data models. When you design your components and services, think about the interfaces they depend on rather than concrete implementations to improve modularity and testability.
How Dependency Injection Relates to factories​
Dependency Injection (DI) and the Factory Method pattern are related but distinct concepts in software design, and they can be used together in certain scenarios.
Dependency Injection​
- Concept: Dependency Injection is a design pattern used to implement Inversion of Control (IoC) between classes and their dependencies. Instead of a class creating dependencies itself, they are provided (injected) from the outside, often by an IoC container or a framework.
- Purpose: The main purpose is to decouple classes from their dependencies, making the system more modular, easier to test, and more adaptable to change.
- Implementation: Dependencies (like service classes, configurations, or resources) are 'injected' into a class through constructors, methods, or directly into fields.
How They Relate and Can Be Used Together​
-
DI Containers Using Factories: Many dependency injection frameworks or containers use some form of factory pattern under the hood to create instances of dependencies. When you ask a DI container for an instance, it may internally use a factory to create this instance, especially if the creation logic is complex.
-
Factories for Complex Dependencies: Sometimes, dependencies themselves require complex setup. In such cases, a factory might be used to encapsulate the creation logic, and the factory itself is injected into the class requiring the dependency.
-
Combination in Configuration: In more complex systems, you might find that factories are used to create specific types of objects based on runtime conditions, and these factories are then injected into classes that need them.
Example in TypeScript​
Imagine a scenario in a TypeScript application where you need a service that can handle different types of data processing. You might have a DataProcessorFactory
that creates different types of DataProcessor
instances. This factory could be injected into a class that needs a DataProcessor
.
interface DataProcessor {
process(data: any): any;
}
class JsonDataProcessor implements DataProcessor {
process(data: any): any {
// Process JSON data
}
}
class XmlDataProcessor implements DataProcessor {
process(data: any): any {
// Process XML data
}
}
class DataProcessorFactory {
createDataProcessor(type: string): DataProcessor {
if (type === 'JSON') {
return new JsonDataProcessor();
} else if (type === 'XML') {
return new XmlDataProcessor();
}
throw new Error('Unsupported data type');
}
}
class DataService {
constructor(private dataProcessorFactory: DataProcessorFactory) {}
processData(data: any, type: string): any {
const processor = this.dataProcessorFactory.createDataProcessor(type);
return processor.process(data);
}
}
In this example, DataService
depends on DataProcessorFactory
, which is injected into it. The factory then creates the specific DataProcessor
needed based on the type of data.
Tip for React Development​
When working with React and managing state or side-effects, dependency injection isn't as common as in backend or more traditional OOP development. However, understanding these principles can still be beneficial for organizing your logic, especially when dealing with contexts or higher-order components. It helps in keeping your components clean, testable, and decoupled from specific logic implementations.
Using Interface​
In the context of the Factory Method design pattern, you don't necessarily need to use inheritance; using an interface is a perfectly valid and often preferred approach, especially in languages that support interfaces well, like TypeScript or Java.
The Factory Method pattern is primarily about providing a way for a class to delegate the creation of its objects to subclasses. This is typically achieved through either inheritance or interfaces. Here's a quick rundown of both approaches:
Using Inheritance​
- Abstract Class: The base class is an abstract class with a defined method (the "factory method") that returns an object of a certain type. This method is typically abstract or virtual, meaning that the subclasses are expected to implement it.
- Concrete Classes: These are subclasses of the abstract class, and they override the factory method to create and return instances of different types.
Using Interfaces​
- Interface: Instead of an abstract class, you define an interface with the factory method. This method is meant to create and return an object of a certain type.
- Implementing Classes: Various classes implement this interface and provide concrete implementations of the factory method, creating and returning specific types of objects.
Comparison and Use Cases​
- Flexibility: Interfaces are generally more flexible than abstract classes because they don't force a class hierarchy. A class can implement multiple interfaces, but it can only inherit from one class (in languages that don't support multiple inheritance).
- Code Reusability: Inheritance allows you to reuse code in the base class, which can be beneficial if there is common logic that all factory methods should follow.
- Design Choice: If you want strict control over the methods and possibly some shared code, inheritance might be the way to go. If you're looking for flexibility and loose coupling, interfaces are preferable.
In languages like TypeScript and Java, interfaces are often used to implement the Factory Method pattern due to their flexibility and the ease of maintaining loosely coupled code. However, the choice between inheritance and interfaces can depend on the specific requirements and constraints of your project.