Prototype
The Prototype pattern is a creational design pattern used in software development. It is particularly useful in scenarios where the cost of creating a new instance of an object is more expensive or complex than copying an existing one. This pattern is especially useful in languages or environments where instances of a class can be cloned.
Here's a brief overview of the Prototype pattern:
Concept​
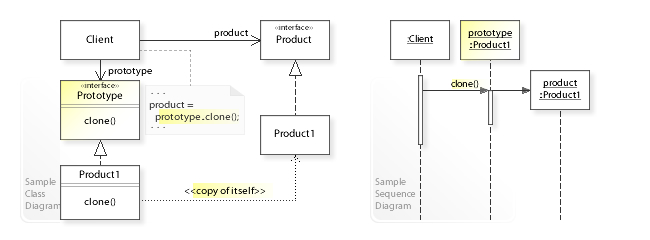
- Prototype: This is the original object that will be cloned.
- Clone: This involves creating a copy of the prototype object. The cloning can be either shallow (copying the values of the fields only) or deep (copying everything, including objects referenced by the fields).
Usage​
- Avoids the overhead of initializing an object: Instead of going through a costly initialization process, an existing object is cloned. This is particularly useful when creating an object is more resource-intensive than copying an existing one.
- Creating objects on the fly: Useful in situations where you need to create objects dynamically at runtime that share certain characteristics.
- Configuring an object with a large number of parameters: A pre-configured prototype can be cloned and slightly modified as needed.
Implementation in TypeScript​
Here's a simple example in TypeScript:
interface Prototype {
clone(): Prototype;
}
class ConcretePrototype implements Prototype {
public field: number;
constructor(field: number) {
this.field = field;
}
// Implementing the clone method
public clone(): this {
// For deep clone, you might need a more complex implementation
return Object.assign(Object.create(Object.getPrototypeOf(this)), this);
}
}
function clientCode() {
const prototype = new ConcretePrototype(100);
const clonePrototype = prototype.clone();
console.log(prototype.field); // Output: 100
console.log(clonePrototype.field); // Output: 100
clonePrototype.field = 200;
console.log(clonePrototype.field); // Output: 200 (independent from the original)
}
clientCode();
In this example, ConcretePrototype
is the class that implements the Prototype interface. It has a clone
method that creates a new object that is a copy of itself.
The Intrinsic relationship between Javascript and the Prototype pattern​
The relationship between JavaScript and the Prototype pattern is intrinsic to the language's design and functionality. JavaScript is a prototype-based language, which means it uses prototypes instead of classes for inheritance and object creation. This is fundamentally different from class-based object-oriented languages like Java or C++.
Key Points of JavaScript's Prototype System:​
-
Prototype Object: Every JavaScript function has a prototype property (except arrow functions), which is an object. Objects created by a constructor function (using the
new
keyword) inherit from the prototype object of the constructor function. -
Prototype Chain: When you try to access a property or method of an object, JavaScript first looks at the object itself. If it doesn't find it there, it looks at the object's prototype, then the prototype's prototype, and so on up the chain until it reaches the Object prototype, which is the top of the prototype chain.
-
Prototype-based Inheritance: Instead of class-based inheritance, JavaScript uses prototype-based inheritance. Objects inherit properties and methods from other objects. You can set an object's prototype to another object, effectively inheriting from it.
Example in JavaScript​
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
return `Hello, my name is ${this.name}`;
};
const person1 = new Person("Alice");
console.log(person1.greet()); // Output: Hello, my name is Alice
// person1 inherits the greet method from Person's prototype.
In this example, Person
is a constructor function with a prototype property. When we create a new instance of Person
, the instance inherits the greet
method from Person.prototype
.
Relationship to the Prototype Pattern​
-
Natural Fit for Prototype Pattern: Since JavaScript inherently uses prototypes, it is a natural fit for the Prototype pattern. You can easily create objects based on prototypes (template objects), and customize or extend them.
-
Flexibility: JavaScript’s prototype-based inheritance provides greater flexibility. It allows you to add or change properties and methods at runtime, affecting all objects that inherit from that prototype.
-
Performance Considerations: Using prototypes can be more memory-efficient, as inherited properties and methods are shared among all instances, rather than duplicated for each instance.
Usage in Fullstack Development​
- Efficiency in Frontend: Prototypes are particularly useful in frontend JavaScript frameworks and libraries, where you might need multiple instances of a component or object with similar functionalities.
- Backend Applications: In Node.js, understanding prototypes is crucial for effectively using and creating modules and middleware.
In summary, JavaScript's prototype-based system is not just an implementation of the Prototype pattern; it is a core aspect of the language's identity and functionality. Understanding prototypes in JavaScript is essential for effective programming, especially when it comes to inheritance and object creation.
Tips for Fullstack Developers​
- When using JavaScript or TypeScript, remember that objects are reference types. Be mindful of shallow and deep cloning.
- The Prototype pattern can be particularly useful in front-end development when you have template components that need to be duplicated with minor variations.
- This pattern can help optimize performance in React applications, especially when dealing with complex state objects that need to be replicated across components.
The Prototype pattern can be a powerful tool in your development arsenal, especially when used appropriately to reduce initialization overhead or when dealing with complex object configurations.