Visitor
The Visitor pattern is a behavioral design pattern used in object-oriented programming. It allows you to add new operations to existing object structures without modifying those structures. This is particularly useful in situations where you have a complex object structure, like a document with various elements (paragraphs, images, etc.), and you want to perform operations on these elements without changing their classes.
Concept of Visitor Patternβ
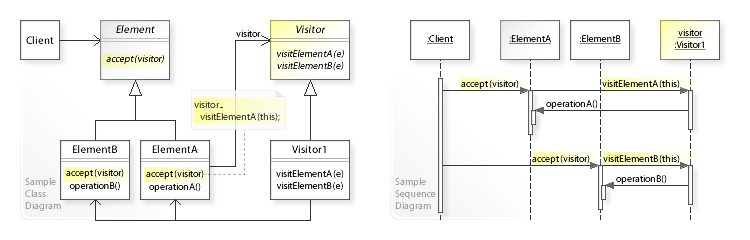
-
Visitor Interface: This defines a
visit
method for each type of element that can be visited. The method's name and signature identify the type of element being visited. -
Concrete Visitor: Implements the visitor interface and defines the operation to be performed on each type of element.
-
Element Interface: Provides an
accept
method that takes a visitor as an argument. -
Concrete Element: Implements the element interface and defines the
accept
method to call thevisit
method on the visitor, passingthis
as an argument. -
Object Structure: A collection of elements that can be iterated over to allow the visitor to visit each element.
Advantagesβ
- Separation of Concerns: Operations on elements are separated from the elements themselves.
- Extensibility: New operations can be added without modifying the object structure.
- Single Responsibility Principle: Each class has a single responsibility.
Disadvantagesβ
- Complexity: Can make the code more complicated, especially if not many operations are added.
- Violation of Encapsulation: Visitors often require access to the private fields and methods of the elements they visit.
Example in TypeScriptβ
interface Visitor {
visitConcreteElementA(element: ConcreteElementA): void;
visitConcreteElementB(element: ConcreteElementB): void;
}
interface Element {
accept(visitor: Visitor): void;
}
class ConcreteElementA implements Element {
accept(visitor: Visitor): void {
visitor.visitConcreteElementA(this);
}
operationA(): string {
return 'ElementA Operation';
}
}
class ConcreteElementB implements Element {
accept(visitor: Visitor): void {
visitor.visitConcreteElementB(this);
}
operationB(): string {
return 'ElementB Operation';
}
}
class ConcreteVisitor implements Visitor {
visitConcreteElementA(element: ConcreteElementA) {
console.log(`${element.operationA()} visited by ConcreteVisitor`);
}
visitConcreteElementB(element: ConcreteElementB) {
console.log(`${element.operationB()} visited by ConcreteVisitor`);
}
}
// Usage
const elements: Element[] = [new ConcreteElementA(), new ConcreteElementB()];
const visitor: Visitor = new ConcreteVisitor();
elements.forEach(e => e.accept(visitor));
In this example, ConcreteVisitor
can perform operations on ConcreteElementA
and ConcreteElementB
without them needing to know the details of these operations.
Tip for Fullstack Developersβ
As a fullstack developer, when designing complex applications with various types of objects needing different processing, consider the Visitor pattern. It's particularly useful in scenarios where objects are relatively stable but operations on them are expected to change or grow over time. Remember to balance the use of this pattern with the complexity it introduces to your codebase.
It's important to recognize scenarios where the Visitor pattern might be useful, especially when dealing with complex data structures or systems requiring flexible operations. However, always weigh the complexity it adds against its benefits. In many web development scenarios, simpler patterns or direct solutions might be more appropriate. The Visitor pattern shines in systems where operations on objects are frequently changing, or you need to separate algorithmic logic from object structure.
Some practical examplesβ
-
Document Object Models (DOM) Manipulation: In complex document structures like HTML or XML, the Visitor pattern can be used to perform operations on various elements without altering their structure. For example, you might have a Visitor that extracts certain types of information from a DOM, like collecting all hyperlinks or modifying specific attributes of certain elements.
-
Compiler Design: In compilers, the Visitor pattern is often used for operations on abstract syntax trees (AST). Different visitor objects can perform syntax checking, code optimization, type checking, or code generation on various nodes of the AST.
-
Graphics Rendering Engines: In a graphic design application, you may have a complex object structure representing graphical elements (like shapes, lines, and text). A Visitor can be used to perform operations like rendering to a canvas, exporting to different formats, or applying transformations without changing the class definitions of these elements.
-
Reporting and Analytics: In scenarios where you have a complex object structure representing business data (like sales data, customer profiles), Visitors can be used to generate different types of reports or perform analytics by iterating over these structures and accumulating results.
-
Networking and Hardware Simulation: In network simulations or hardware emulation, the Visitor pattern can be used to interact with different types of network nodes or hardware components. Each component can accept different visitors for operations like data transmission, status checking, or diagnostics.
-
Game Development: In game engines, the Visitor pattern can be used for operations on game objects, like collision detection, rendering, or AI processing. Each type of game object (characters, terrains, items) can be visited and processed differently.
-
Insurance Systems: In insurance software systems, you might have different policies and rules. A Visitor can be used to calculate premiums or benefits for different types of insurances without embedding these calculations within the insurance class hierarchy.
-
Plugin Systems: If you have a system that supports plugins or extensions, the Visitor pattern can allow these plugins to interact with or modify existing objects or data structures within the system without needing to alter the core system's code.
Visitor Pattern and Recursionβ
The Visitor pattern is commonly used in scenarios involving recursive traversal of a composite structure, like trees or graphs. Here's why it's a good fit:
-
Recursive Data Structures: In structures like trees (e.g., binary trees, abstract syntax trees in compilers, or DOM trees in web development), each node might have a similar set of operations to be performed, but the specific action might depend on the node's type.
-
Decoupling Operations from Structures: Visitor pattern allows operations to be defined separately from the nodes' classes. This is particularly useful in recursive structures, as it separates the logic of traversal from the operations performed at each node.
-
Flexibility in Adding New Operations: With recursive structures, you often need to add new operations without changing the structure itself. The Visitor pattern allows these new operations to be added easily, respecting the Open/Closed principle.
Example with Recursionβ
Consider a simple binary tree. Each node in the tree could accept a visitor, and the visitor's method could be called recursively for each child of the node.
Here's a basic TypeScript example:
// Visitor interface
interface Visitor {
visitNode(node: TreeNode): void;
}
// Tree node
class TreeNode {
constructor(public value: number, public left?: TreeNode, public right?: TreeNode) {}
accept(visitor: Visitor): void {
// Visit current node
visitor.visitNode(this);
// Recursively visit left and right children
if (this.left) {
this.left.accept(visitor);
}
if (this.right) {
this.right.accept(visitor);
}
}
}
// Concrete visitor
class ConcreteVisitor implements Visitor {
visitNode(node: TreeNode): void {
console.log(`Visiting node with value: ${node.value}`);
}
}
// Example usage
const tree = new TreeNode(1, new TreeNode(2), new TreeNode(3));
const visitor = new ConcreteVisitor();
tree.accept(visitor);
In this example, each TreeNode
can accept a Visitor
. The accept
method in TreeNode
is where recursion happensβit calls accept
on its left and right children, thus traversing the entire tree. The ConcreteVisitor
implements an operation to be performed on each TreeNode
.
Tips for Fullstack Developers:β
-
Understand Your Data Structures: If you're working with recursive data structures, consider how design patterns like the Visitor can help manage complexity and enhance maintainability.
-
Balance Between Pattern and Simplicity: While the Visitor pattern provides a structured way to handle complex scenarios, always balance the use of design patterns with the overall simplicity and readability of your code.
-
Think About Extensibility: If your application might require new operations on recursive structures in the future, a pattern like Visitor could be a good fit, as it allows adding new operations without modifying existing classes.
Understanding when and how to effectively use design patterns like the Visitor pattern, especially in the context of recursive structures, can significantly impact the maintainability and scalability of your code, particularly in complex applications.
FP implementationβ
Creating a functional programming (FP) implementation of the Visitor pattern in TypeScript can be a bit challenging since FP generally eschews the kind of type hierarchy and polymorphism that's central to the Visitor pattern in object-oriented programming. However, you can use features like first-class functions and higher-order functions to achieve similar behavior in a more functional style.
Here's a TypeScript example of how you might implement a Visitor-like pattern using functional programming concepts:
// Define a type for each kind of element
type ElementA = { type: "A"; data: string };
type ElementB = { type: "B"; data: number };
// Define a union type for all elements
type Element = ElementA | ElementB;
// Define a function for each kind of visitor operation
const visitElementA = (element: ElementA) => `Processing ElementA: ${element.data}`;
const visitElementB = (element: ElementB) => `Processing ElementB: ${element.data}`;
// Define a function that takes an element and decides which visitor function to apply
const applyVisitor = (element: Element) => {
switch (element.type) {
case "A":
return visitElementA(element);
case "B":
return visitElementB(element);
}
};
// Example usage
const elements: Element[] = [{ type: "A", data: "Hello" }, { type: "B", data: 42 }];
elements.map(applyVisitor).forEach(result => console.log(result));
In this FP-style implementation:
- Each
Element
type (ElementA
,ElementB
) is a distinct type with atype
field to discriminate between them. - There are separate functions (
visitElementA
,visitElementB
) to handle each element type. - The
applyVisitor
function acts as a dispatcher. It decides which function to apply to each element based on its type.
Remember, while design patterns like Visitor have their roots in object-oriented programming, the underlying principles can often be adapted to a functional programming style, especially in TypeScript, which offers features from both paradigms.