State
The State Pattern is a behavioral design pattern that allows an object to alter its behavior when its internal state changes. This pattern is particularly useful in situations where the behavior of an object depends on its state, and it needs to change its behavior at runtime depending on that state.
In the context of the State Pattern, the state-specific behavior is encapsulated in separate state objects. The main object, often referred to as the context, holds a reference to a state object that represents its current state. When the context needs to perform some behavior, it delegates it to the current state object. As the state of the context changes, it switches the state object it holds to a different one that represents its new state.
Key Conceptsβ
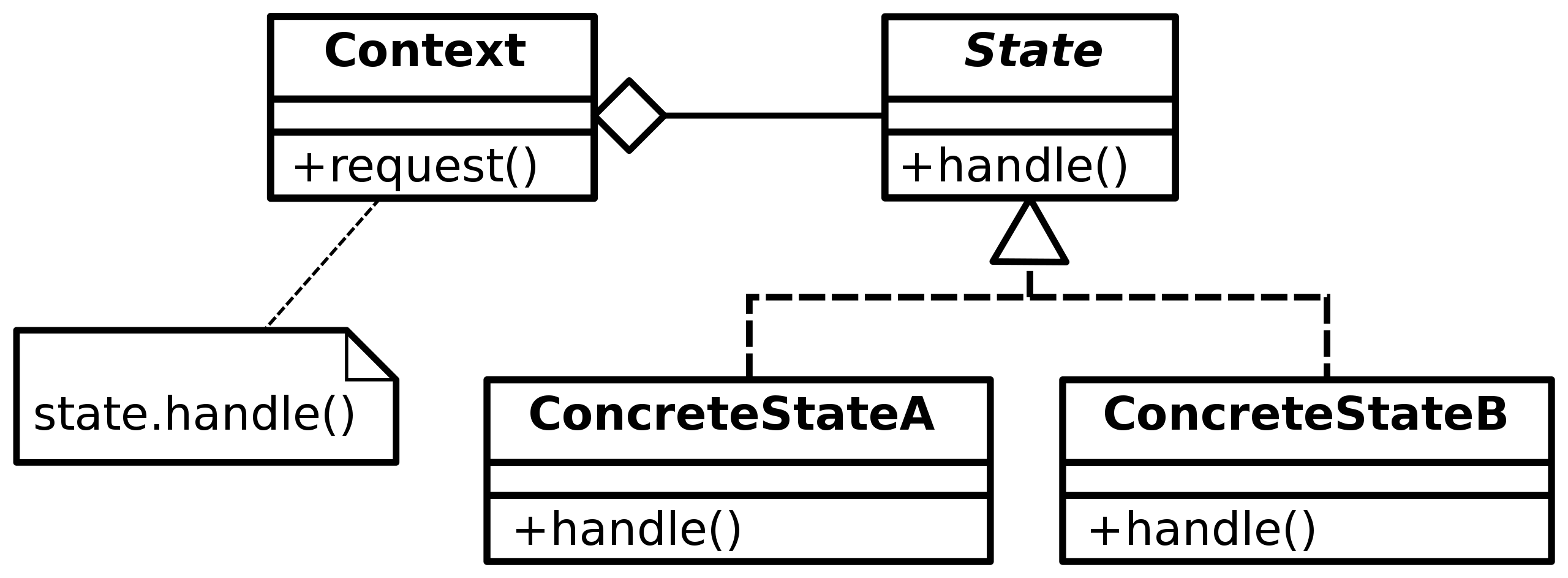
State Pattern in the Wikipedia
-
Context: The primary object whose behavior varies according to its internal state. It maintains a reference to a concrete state object that represents its current state.
-
State Interface: An interface or abstract class defining the methods that each concrete state should implement.
-
Concrete States: Classes that implement the State interface and provide the specific behaviors associated with a state of the Context.
-
Transitions: The Context changes its state by switching the state object it refers to. These transitions can be triggered by events or conditions within the Context or its states.
Benefitsβ
- Single Responsibility Principle: Each state can be in its own class, encapsulating the behavior associated with that state.
- Open/Closed Principle: Itβs easy to introduce new states without changing the context.
- Eliminates Conditional Statements: Reduces complex conditional logic by encapsulating state-specific behaviors into separate classes.
Drawbacksβ
- Increased Number of Classes: For every state, a new class is required, which can increase the complexity of the codebase.
- Potential for Overuse: For simple state changes, using this pattern might be an overkill.
Example in TypeScriptβ
Here's a simple example in TypeScript demonstrating the State Pattern:
// State interface
interface State {
handle(context: Context): void;
}
// Concrete states
class ConcreteStateA implements State {
handle(context: Context): void {
console.log('State A is handling the request.');
context.state = new ConcreteStateB();
}
}
class ConcreteStateB implements State {
handle(context: Context): void {
console.log('State B is handling the request.');
context.state = new ConcreteStateA();
}
}
// Context
class Context {
state: State;
constructor(state: State) {
this.state = state;
}
request(): void {
this.state.handle(this);
}
}
// Usage
const context = new Context(new ConcreteStateA());
context.request(); // State A handles and changes to State B
context.request(); // State B handles and changes to State A
In this example, Context
has a method request()
that delegates the handling to its current state
. The concrete state classes ConcreteStateA
and ConcreteStateB
change the state of the context when handling a request.
Tip for Fullstack Developersβ
As a fullstack developer working mainly with React, you can use the State Pattern to manage complex component states. Instead of having a large switch-case or if-else structure in your components, you can encapsulate the state-related logic in separate classes, making your React components cleaner and easier to maintain.
State Pattern vs Routing in Web Applicationsβ
The State Pattern does have similarities with routing in a web application, especially in the way different states or routes lead to different components or views. Let's explore this comparison:
-
Modularity and Encapsulation:
- State Pattern: Each state is encapsulated in its own class, handling the specific logic and behavior of that state.
- Routing: Each route is typically associated with a specific component or view. This encapsulates the logic and UI for that route.
-
Change of State/Route:
- State Pattern: The context (main object) changes its state by switching the state object it refers to.
- Routing: The application changes its view by switching the route, often triggered by user navigation (like clicking a link or a button).
-
Delegation of Behavior:
- State Pattern: The context delegates behavior to the current state object.
- Routing: The router delegates the rendering of the content to the component associated with the current route.
-
Dynamic Behavior:
- State Pattern: The context's behavior changes dynamically based on its internal state.
- Routing: The displayed content and behavior of the application change dynamically based on the current route.
Example in a React Router Contextβ
In a React application with routing (using React Router, for example), each route can be seen as a 'state', and the components rendered by these routes can be seen as the behavior specific to that state.
import React from 'react';
import { BrowserRouter as Router, Route, Switch, Link } from 'react-router-dom';
const Home = () => <div>Home View</div>;
const About = () => <div>About View</div>;
const Contact = () => <div>Contact View</div>;
const App = () => {
return (
<Router>
<div>
<nav>
<Link to="/">Home</Link>
<Link to="/about">About</Link>
<Link to="/contact">Contact</Link>
</nav>
<Switch>
<Route path="/about">
<About />
</Route>
<Route path="/contact">
<Contact />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
</div>
</Router>
);
}
export default App;
In this example, each route (/
, /about
, /contact
) represents a different 'state' of the application, and each associated component (Home
, About
, Contact
) represents the behavior and view for that state.
Development Tipβ
Understanding this analogy can help you design more organized and modular React applications. Just like states in the State Pattern, you can think of routes as distinct states of your app, each managing its own behavior and presentation. This approach can lead to a clearer separation of concerns, making your application easier to maintain and scale.