Interpreter
The Interpreter pattern is a behavioral design pattern used in software engineering, particularly useful when developing domain-specific languages or parsers. It provides a way to evaluate sentences in a language by defining a representational grammar and then interpreting sentences using this grammar.
Here's a general overview of how the Interpreter pattern works:
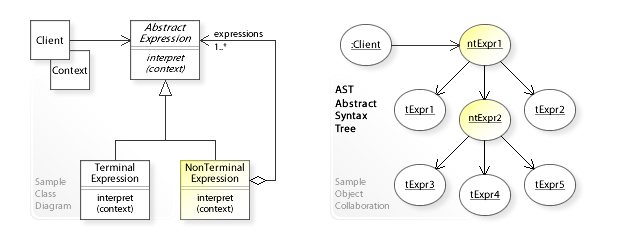
Interpreter pattern in the Wikpedia
-
Abstract Expression: This is an interface that declares an interpret method. It's common for all nodes (expressions) in the abstract syntax tree (AST).
-
Terminal Expression: These classes implement the Abstract Expression interface for terminal symbols in the grammar. Terminal expressions don't have any sub-expressions.
-
Non-terminal Expression: Also implementing the Abstract Expression interface, these classes represent non-terminal symbols in the grammar. Non-terminal expressions typically have one or more expressions as sub-expressions.
-
Context: This object contains information that's global to the interpreter. It's often used to store state or additional information needed by expressions during interpretation.
-
Client: The client builds the abstract syntax tree representing a particular sentence in the language that the grammar defines. The tree is assembled from instances of the Non-terminal Expression and Terminal Expression classes.
In a real-world application, the Interpreter pattern can be complex, especially for robust grammars. It's best suited for simple languages or specific types of parsing where the grammar is limited and manageable.
Regarding TypeScript implementation, the Interpreter pattern would involve defining interfaces and classes corresponding to the abstract and concrete expressions. The interpret method would be implemented in each concrete class to handle the specific logic of interpreting that part of the grammar.
Useful scenarios​
The Interpreter pattern is particularly useful in scenarios where a program needs to interpret or evaluate sentences in a language according to a defined grammar. Here are some of the most common scenarios where the Interpreter pattern is used:
-
Domain-Specific Languages (DSLs): When creating a DSL for a specific problem domain, the Interpreter pattern can be used to parse and interpret the language. This is common in areas like configuration scripts, simple query languages, or specialized scripting within software applications.
-
Expression Evaluation: In scenarios where a system needs to evaluate mathematical, logical, or other types of expressions dynamically, the Interpreter pattern can be a good fit. Examples include calculators, rule engines, or decision-making systems.
-
Programming Language Compilers and Interpreters: While a full-fledged compiler or interpreter for a programming language is typically more complex, the Interpreter pattern can be a foundational principle for implementing certain aspects of these systems, such as parsing and executing simple syntax structures.
-
SQL Parsing: In database systems, interpreting SQL queries involves parsing and understanding SQL syntax. The Interpreter pattern can be used to implement parts of the SQL parsing process.
-
Regular Expressions: Regular expression engines often use a form of the Interpreter pattern to parse and match text based on a pattern. This is a classic example of interpreting a language (regular expressions) to perform operations (text matching and extraction).
-
Configuration File Parsing: Many applications use configuration files with a specific syntax. The Interpreter pattern can be used to parse and interpret these files to extract settings and configurations.
-
Natural Language Processing (NLP): Simple forms of NLP, like parsing and understanding basic commands or queries, can be implemented using the Interpreter pattern. This is more common in cases where the language scope is limited and well-defined.
-
Template Engines: In web and application development, template engines that interpret and render templates with embedded tags or expressions often use principles similar to the Interpreter pattern.
Basic Example​
Imagine a scenario where we want to interpret simple expressions consisting of addition and subtraction of numbers.
In this example, we'll define the following:
Expression
- An abstract expression interface.NumberExpression
- A terminal expression for numbers.AddExpression
andSubtractExpression
- Non-terminal expressions for addition and subtraction.Context
is not needed in this simple example, as our expressions are self-contained.- The client will create and interpret these expressions.
First, define the Expression
interface:
interface Expression {
interpret(): number;
}
Now, implement the terminal expression for numbers:
class NumberExpression implements Expression {
private value: number;
constructor(value: number) {
this.value = value;
}
interpret(): number {
return this.value;
}
}
Next, implement the non-terminal expressions for addition and subtraction:
class AddExpression implements Expression {
private leftExpression: Expression;
private rightExpression: Expression;
constructor(left: Expression, right: Expression) {
this.leftExpression = left;
this.rightExpression = right;
}
interpret(): number {
return this.leftExpression.interpret() + this.rightExpression.interpret();
}
}
class SubtractExpression implements Expression {
private leftExpression: Expression;
private rightExpression: Expression;
constructor(left: Expression, right: Expression) {
this.leftExpression = left;
this.rightExpression = right;
}
interpret(): number {
return this.leftExpression.interpret() - this.rightExpression.interpret();
}
}
Finally, the client code that uses these classes could look like this:
// Creating an expression (1 + (2 - 3))
const expression: Expression = new AddExpression(
new NumberExpression(1),
new SubtractExpression(
new NumberExpression(2),
new NumberExpression(3)
)
);
// Interpreting the expression
const result = expression.interpret();
console.log(result); // Output will be 0
In this example, the interpret()
method in each concrete class (NumberExpression
, AddExpression
, SubtractExpression
) implements how to interpret that part of the expression. The client creates an abstract syntax tree representing the expression and then interprets it.
Template Engine Example​
This template engine will interpret custom tags in a string and replace them with provided values. For simplicity, our template tags will be enclosed in curly braces, like {tag}
.
In this example, we'll have:
Expression
- An interface for expressions in our template language.LiteralExpression
- A terminal expression for plain text.TagExpression
- A terminal expression for tags.Template
- A class that will parse a template string into expressions and interpret it by replacing tags with values.
Here's how we can implement it:
1. Define the Expression
Interface:​
interface Expression {
interpret(context: Map<string, string>): string;
}
2. Implement the LiteralExpression
for Plain Text:​
class LiteralExpression implements Expression {
private text: string;
constructor(text: string) {
this.text = text;
}
interpret(context: Map<string, string>): string {
return this.text;
}
}
3. Implement the TagExpression
for Tags:​
class TagExpression implements Expression {
private tag: string;
constructor(tag: string) {
this.tag = tag;
}
interpret(context: Map<string, string>): string {
return context.get(this.tag) || '';
}
}
4. Implement the Template
Class:​
class Template {
private expressions: Expression[];
constructor(template: string) {
this.expressions = [];
this.parse(template);
}
private parse(template: string): void {
const regex = /{([^}]+)}/g;
let lastIndex = 0;
let match;
while ((match = regex.exec(template)) !== null) {
if (match.index > lastIndex) {
this.expressions.push(new LiteralExpression(template.slice(lastIndex, match.index)));
}
this.expressions.push(new TagExpression(match[1]));
lastIndex = regex.lastIndex;
}
if (lastIndex < template.length) {
this.expressions.push(new LiteralExpression(template.substr(lastIndex)));
}
}
interpret(context: Map<string, string>): string {
return this.expressions.map(expr => expr.interpret(context)).join('');
}
}
5. Using the Template Engine:​
const templateString = "Hello, {name}! Welcome to {place}.";
const template = new Template(templateString);
const context = new Map<string, string>([
['name', 'John'],
['place', 'the Internet']
]);
const result = template.interpret(context);
console.log(result); // Output: "Hello, John! Welcome to the Internet."
In this example, Template
parses a template string into a series of LiteralExpression
and TagExpression
objects. When interpreting the template, it replaces each TagExpression
with its corresponding value from the context map.
Considerations​
- Leverage TypeScript's type system to ensure the correctness of your expressions and context data.
- When integrating with a frontend framework like React, consider how your template engine might fit into the component lifecycle and state management.
- For more complex scenarios, you might want to extend the functionality of your template engine with features like conditional rendering, loops, or custom filters. TypeScript's class and interface system can help you manage this complexity effectively.
TypeScript Tips​
- Leverage TypeScript's support for object-oriented concepts to structure the different parts of the interpreter in a clear, maintainable way.
- When considering the Interpreter pattern, evaluate whether the complexity it introduces is justified for your scenario. For complex languages, more advanced parsing techniques or existing libraries might be more appropriate.
- In the context of full-stack development, be mindful of performance implications, especially if interpretation happens on the client side (in a web browser). The Interpreter pattern can be resource-intensive.
- If you're working with React or other frontend frameworks, consider how the Interpreter pattern might interact with your UI rendering logic, particularly if you're interpreting template languages or DSLs for UI components.