Observer
The Observer Pattern falls under the category of Behavioral Design Patterns. It's particularly useful in web development for managing how different parts of your application communicate and react to changes. Let's break it down.
In a nutshell, the Observer Pattern is about creating a one-to-many dependency for disseminating state changes, making it incredibly useful in dynamic and interactive web applications.
Concept​
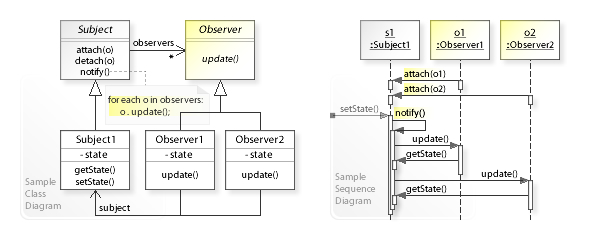
- Purpose: The Observer Pattern is used to create a one-to-many dependency between objects. This means when one object changes its state, all its dependents are notified and updated automatically.
- Components:
- Subject: The main object that holds the state. It notifies observers about changes.
- Observers: These are the objects that want to be informed about the state changes in the subject.
How It Works​
- Registering Observers: Observers subscribe to the subject to receive updates.
- State Change in Subject: Whenever the subject undergoes a change (e.g., user input, data retrieval), it updates its state.
- Notification: The subject then notifies all registered observers about this change.
- Reaction: Each observer, upon receiving the notification, reacts accordingly (e.g., UI update, data processing).
Use Cases in Web Development​
- Event Handling: For example, in a React application, components can observe certain state variables and re-render when these variables change.
- Model-View-Controller (MVC) Framework: The model is the subject, and views are the observers. When the model updates (like database changes), views react (UI updates).
- Redux: Here, the Redux store is the subject, and React components are observers. Components subscribe to store changes and update when state changes.
Advantages​
- Loose Coupling: The subject doesn't need to know the specifics about observers. This makes the system components more independent and easier to extend.
- Dynamic Subscription: Objects can subscribe or unsubscribe from notifications dynamically during runtime.
Considerations​
- Memory Leaks: In web frameworks like React, not unsubscribing from observers (like event listeners or state subscriptions) can lead to memory leaks.
- Over-notification: Sometimes, observers may be notified too often, leading to performance issues.
Implementation in JavaScript​
class Subject {
constructor() {
this.observers = [];
}
subscribe(observer) {
this.observers.push(observer);
}
unsubscribe(observer) {
this.observers = this.observers.filter(obs => obs !== observer);
}
notify(data) {
this.observers.forEach(observer => observer.update(data));
}
}
class Observer {
update(data) {
console.log("Observer notified with data:", data);
}
}
// Usage
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.subscribe(observer1);
subject.subscribe(observer2);
subject.notify("Hello World!"); // Notifies all observers
FP example​
Implementing the Observer Pattern in a functional programming (FP) style in JavaScript can be interesting since FP emphasizes immutability and stateless functions.
This implementation respects FP principles as much as possible while implementing the Observer Pattern, which inherently requires managing state and dealing with side effects.
Functional Approach to Observer Pattern​
- Create Observers: Observers are simple functions that do something with the data they receive.
- Subject (Observable): The subject will be a function that holds the state and a list of observers. It will have methods to subscribe or unsubscribe observers and to notify them.
// Observer function
const observer = data => {
console.log("Observer received data:", data);
};
// Creates an observable
const createObservable = () => {
let observers = [];
return {
subscribe: (observerFunction) => {
observers.push(observerFunction);
},
unsubscribe: (observerFunction) => {
observers = observers.filter(obs => obs !== observerFunction);
},
notify: (data) => {
observers.forEach(observerFunction => observerFunction(data));
}
};
};
// Usage
const observable = createObservable();
observable.subscribe(observer);
observable.notify('Hello FP World!'); // This will log: "Observer received data: Hello FP World!"
observable.unsubscribe(observer);
observable.notify('This will not be logged');
Explanation​
- Observers: Here, observers are just functions. In the example,
observer
is a function that logs the received data. - createObservable: This function creates an observable. The observable has
subscribe
,unsubscribe
, andnotify
methods. It maintains a list of observer functions. - Immutability: We've respected FP principles by not modifying the observers array directly for unsubscribing. Instead, we're creating a new array without the unsubscribed observer.
Characteristics​
- State and Side Effects: While FP prefers stateless and pure functions, the observer pattern inherently requires some state (the list of observers) and side effects (notifying observers).
- Immutability: The pattern is adapted to FP by avoiding direct mutation where possible (like in the
unsubscribe
method).
Most common partners in crime​
The most common collaborations with the Observer Pattern in web development are:
-
MVC (Model-View-Controller) Framework:
- This is perhaps the most prevalent use of the Observer Pattern. The Model acts as the subject, and Views act as observers. When the Model changes (due to business logic or user input), it notifies the Views, which then update themselves accordingly.
-
Singleton Pattern:
- Often, the subject in an Observer Pattern is implemented as a Singleton, particularly in scenarios where a centralized management of state or events is needed, like a global event manager or a central state in an application (e.g., Redux store in a React application).
-
State Pattern:
- The State Pattern is frequently used together with the Observer Pattern to manage state changes in a more organized manner. The subject's state changes can trigger different behaviors in the observers, making the system more dynamic and flexible.
These combinations are especially common in web development and software design because they offer a robust framework for managing state and interactions in complex applications. They help in building scalable, maintainable, and efficient software systems.