Iterator
The Iterator Pattern is a behavioural design pattern used in object-oriented programming to provide a way to access the elements of an aggregate object sequentially without exposing its underlying representation. It's a part of the Gang of Four design patterns and is particularly useful in programming languages that support object-oriented programming, like TypeScript.
Key Concepts of Iterator Pattern​
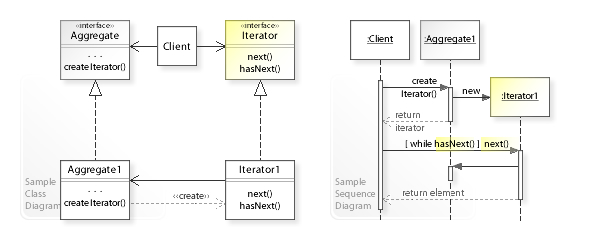
-
Iterator Interface: This defines the standard operations like
next()
,hasNext()
, and sometimesreset()
which are essential for traversal. -
Concrete Iterator: Implements the iterator interface and is responsible for managing the current position of the iterator.
-
Aggregate Interface: This provides a way to create an iterator object to traverse its elements.
-
Concrete Aggregate: Implements the aggregate interface and returns a concrete iterator for its collection.
Benefits​
- Encapsulation: It hides the internal structure of the data collection.
- Single Responsibility Principle: It separates the logic of iteration from the collection.
- Flexibility and Reusability: It allows traversing different data structures in a uniform way.
Use in TypeScript​
In TypeScript, you can implement the Iterator pattern by defining interfaces for the Iterator and Aggregate, and then implementing these interfaces in concrete classes.
Here is a simple example in TypeScript:
interface Iterator<T> {
next(): T;
hasNext(): boolean;
}
interface Aggregate<T> {
getIterator(): Iterator<T>;
}
class ConcreteIterator<T> implements Iterator<T> {
private collection: T[];
private position: number = 0;
constructor(collection: T[]) {
this.collection = collection;
}
public next(): T {
return this.collection[this.position++];
}
public hasNext(): boolean {
return this.position < this.collection.length;
}
}
class ConcreteAggregate<T> implements Aggregate<T> {
private collection: T[];
constructor(collection: T[]) {
this.collection = collection;
}
public getIterator(): Iterator<T> {
return new ConcreteIterator(this.collection);
}
}
// Usage
const collection = new ConcreteAggregate<number>([1, 2, 3, 4]);
const iterator = collection.getIterator();
while (iterator.hasNext()) {
console.log(iterator.next());
}
This example shows a basic implementation of the Iterator pattern where ConcreteIterator
and ConcreteAggregate
are concrete implementations of Iterator
and Aggregate
interfaces respectively.
FP implementation​
Implementing the Iterator Pattern in a functional programming (FP) style in TypeScript can be an interesting exercise. FP focuses on using pure functions, avoiding shared state, mutable data, and side-effects. TypeScript, being a superset of JavaScript, is well-suited for this style due to its first-class functions and strong typing system.
Here's an example of how you might implement a simple iterator in a functional style:
type Iterator<T> = {
next: () => T | undefined;
};
const createIterator = <T>(items: T[]): Iterator<T> => {
let index = 0;
return {
next: () => {
return index < items.length ? items[index++] : undefined;
}
};
};
// Usage
const numbers = [1, 2, 3, 4, 5];
const iterator = createIterator(numbers);
let item = iterator.next();
while (item !== undefined) {
console.log(item);
item = iterator.next();
}
In this implementation:
-
Type Definition: The
Iterator<T>
type defines a structure for iterators with anext
function that returns the next item orundefined
. -
Factory Function:
createIterator
is a factory function that takes an array and returns an iterator for it. This encapsulates the index and avoids external modification. -
Immutability and Side-Effects: The function maintains immutability and has no side effects outside its scope. The state (
index
) is encapsulated within the function's closure. -
Usage: The iterator is used in a
while
loop to traverse the array.
This functional approach provides a clean and concise way to implement the iterator pattern, emphasizing immutability and avoiding side-effects, which are core principles of functional programming.
Compared to Functors​
The Iterator Pattern and functors, particularly in the context of their map
methods, share some conceptual similarities but also have distinct differences. Let's explore their relationship:
Functor and map
Method​
-
Purpose: A functor, in programming (especially functional programming), is a construct that can be mapped over, meaning you can apply a function to each value it holds, producing a new functor.
-
How It Works: The
map
method takes a function and applies it to each element in the functor (like an array), returning a new functor containing the transformed elements. -
Usage: It's specifically used for applying a transformation to each element, encapsulating the logic of iteration and transformation within the
map
method. -
Control Over Iteration: The client has less control over the iteration process. The
map
method abstracts the iteration, applying the given function to each element automatically and in order.
Relationship and Differences​
-
Similarity: Both patterns deal with processing elements of a collection. The Iterator Pattern allows you to iterate and perform operations, while functors with a
map
method let you transform each element of a collection. -
Difference in Control: With iterators, you have more granular control over the iteration process, whereas with functors and
map
, the focus is on what happens to each element rather than how you iterate over them. -
Transformation vs. Iteration: The key distinction lies in the intent. The Iterator Pattern is about iterating over elements, which might involve various operations, including transformation. On the other hand, functors with
map
are specifically about transforming all elements in a consistent way. -
Abstraction Level:
map
in functors abstracts away the iteration process, focusing on applying a function to each element. The Iterator Pattern is more about manually handling the iteration, providing flexibility in how you interact with each element.
In summary, while both deal with collections and their elements, the Iterator Pattern is about controlling the iteration process, and functors with map
are about consistently applying a transformation to each element in a collection.
Tips for Fullstack Developers​
-
Use with Collections: The iterator pattern is especially useful when dealing with collections in both frontend and backend code.
-
Frameworks and Libraries: Many JavaScript/TypeScript frameworks and libraries, like React, may have built-in iterator patterns (like JavaScript's
Array.prototype.map
orfor...of
loops), so it's good to be familiar with these. -
Performance Considerations: For large datasets, consider the performance implications of iteration and look for more efficient data structures or iteration methods if necessary.
-
TypeScript Advantages: Utilize TypeScript's strong typing to ensure that your iterators work with specific types, adding an extra layer of reliability to your code.