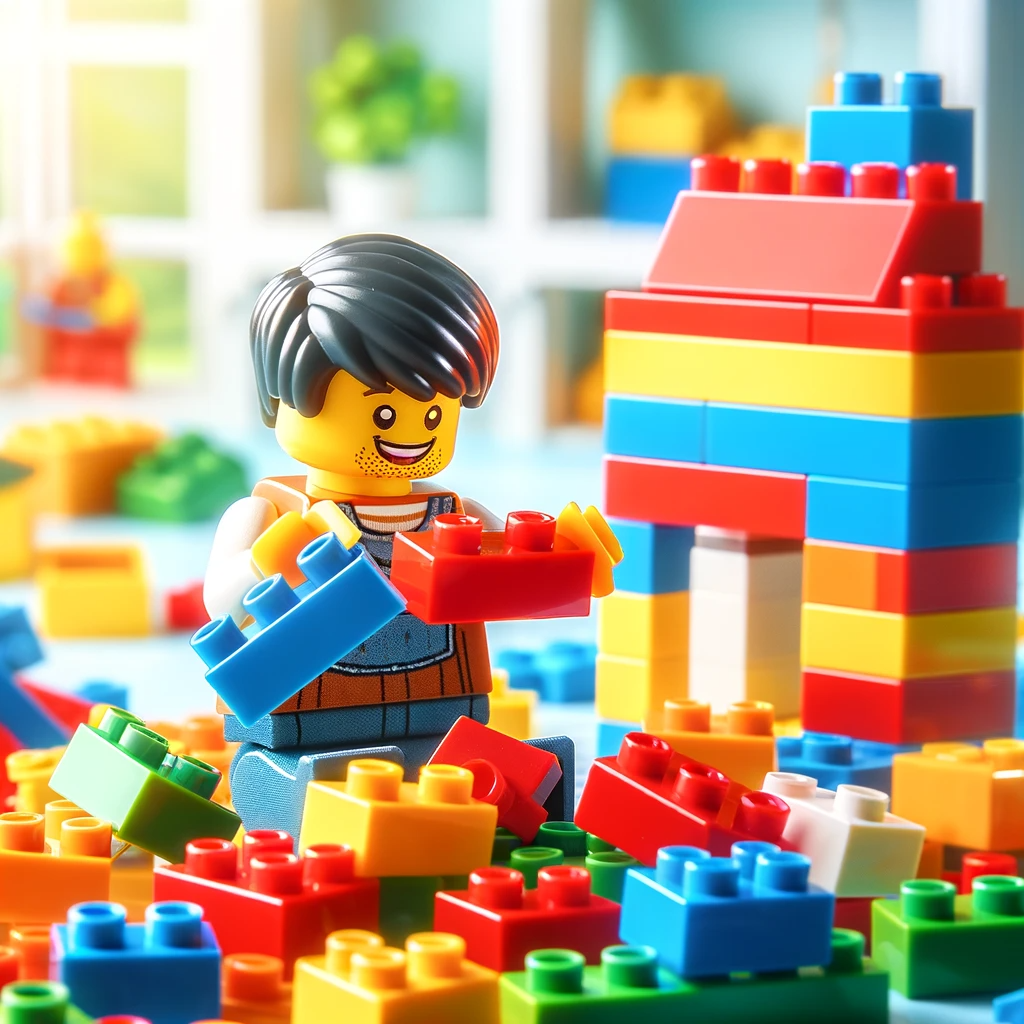
Mixins are a concept in object-oriented programming where a class can include the properties and methods of another class without extending it. Essentially, it's a way to add functionality to a class from multiple sources.
Especially working with modern JavaScript or TypeScript, you might encounter mixins in legacy code or specific libraries but will likely use more contemporary patterns for code reuse and composition.
Mixins are a form of object composition. Instead of inheriting from a single class, an object or class can combine behaviors and attributes from multiple sources. They allow for the reuse of a set of behaviors across various classes. They provide a flexible way to add functionality to a class without using inheritance.
In languages that don't natively support multiple inheritance, mixins can provide a way to gather functionality from multiple sources.
Examplesโ
Old fashionedโ
// Define a mixin object with shared methods
const AnimalMixin = {
eat(amount) {
console.log(`${this.name} is eating ${amount} units of food.`);
this.energy += amount;
},
sleep(hours) {
console.log(`${this.name} is sleeping for ${hours} hours.`);
this.energy += hours * 5;
}
};
// A function to apply the mixin to any constructor's prototype
function applyMixin(constructor, mixin) {
Object.assign(constructor.prototype, mixin);
}
// Define a Dog constructor
function Dog(name, energy) {
this.name = name;
this.energy = energy || 100;
}
// Define a Cat constructor
function Cat(name, energy) {
this.name = name;
this.energy = energy || 100;
}
// Applying the mixin to Dog and Cat prototypes
applyMixin(Dog, AnimalMixin);
applyMixin(Cat, AnimalMixin);
// Creating instances of Dog and Cat
const myDog = new Dog('Buddy');
const myCat = new Cat('Whiskers');
// Using the mixed in methods
myDog.eat(20); // Buddy is eating 20 units of food.
myDog.sleep(5); // Buddy is sleeping for 5 hours.
myCat.eat(15); // Whiskers is eating 15 units of food.
myCat.sleep(3); // Whiskers is sleeping for 3 hours.
Modern mixinโ
// Define a function that takes a base class and returns a new class extended with the mixin
const AnimalMixin = (Base) => class extends Base {
eat(amount) {
console.log(`${this.name} is eating ${amount} units of food.`);
this.energy += amount;
}
sleep(hours) {
console.log(`${this.name} is sleeping for ${hours} hours.`);
this.energy += hours * 5;
}
};
// Define a base Animal class
class Animal {
constructor(name, energy) {
this.name = name;
this.energy = energy || 100;
}
}
// Applying the mixin to create new classes
class Dog extends AnimalMixin(Animal) {}
class Cat extends AnimalMixin(Animal) {}
// Creating instances of Dog and Cat
const myDog = new Dog('Buddy', 100);
const myCat = new Cat('Whiskers', 100);
// Using the mixed-in methods
myDog.eat(20); // Buddy is eating 20 units of food.
myDog.sleep(5); // Buddy is sleeping for 5 hours.
myCat.eat(15); // Whiskers is eating 15 units of food.
myCat.sleep(3); // Whiskers is sleeping for 3 hours.
Considerationsโ
- Complexity: Overuse of mixins can lead to complex and hard-to-understand code structures.
- Namespace Collisions: Functions or properties in a mixin might clash with those in the main class or with other mixins.
- Indirect Structure: It can be harder to understand the overall structure of the program as the inheritance is not straightforward.
Historyโ
Mixins were quite popular in the early days of JavaScript frameworks, especially before ES6 brought classes and modules as they offered a way to add functionality to constructors and objects.
With the introduction of ES6 classes and modules, the use of mixins has declined. Modern JavaScript favors composition and class inheritance, which are more structured and less error-prone.
Still in Use: Despite the decline, mixins are still used in some JavaScript libraries and frameworks. For instance, they are quite prominent in Vue.js for creating reusable component features.
Modern Contextโ
- React: In React, the use of mixins has largely been replaced by higher-order components, render props, and hooks.
- Vue: Vue.js still uses mixins for sharing functionality between components, though the Composition API introduced in Vue 3 offers an alternative.
- TypeScript: TypeScript doesn't support mixins natively, but they can be implemented by intersecting types or using decorators.
Tipsโ
When working with TypeScript, consider using interfaces and types for composition over mixins.
Familiarize yourself with modern alternatives in the frameworks you use, such as hooks in React or the Composition API in Vue.js, which can often achieve what mixins do, but more transparently and with better type support.
Always prefer composition over inheritance where possible for better modularity and reusability.
How to refactor mixinsโ
- Module Pattern: When you want to encapsulate functionality and expose a clear interface.
- Factory Functions: When you need multiple instances of an object with similar properties and methods.
- Composition Functions: When you want the flexibility of mixins without direct inheritance or the fragility of object mutation.
- Utility Libraries: For broad reuse of small, stateless functions across your application.
Composition functionโ
A composition function in JavaScript is a way to combine multiple behaviors or functionalities into a single object without using classical inheritance. Here's a simple example to demonstrate how you might compose an object that has various behaviors using composition functions.
Let's assume you want to create an object that represents a user. This user object needs to have the ability to eat, sleep, and work. Instead of creating a single class or using mixins, you will create functions for each capability and then compose them together.
Here's how you can do it:
// Define a function for each behavior
// Eating behavior
function canEat(state) {
return {
eat: function(food) {
console.log(`Eating ${food}!`);
state.energy += 10;
}
};
}
// Sleeping behavior
function canSleep(state) {
return {
sleep: function(hours) {
console.log(`Sleeping for ${hours} hours!`);
state.energy += hours * 5;
}
};
}
// Working behavior
function canWork(state) {
return {
work: function(hours) {
console.log(`Working for ${hours} hours!`);
state.energy -= hours * 5;
}
};
}
// Compose the object with all behaviors
function createUser(name) {
let state = {
name: name,
energy: 100 // Initial energy level
};
// Use object spread to combine functionalities
return {
...state,
...canEat(state),
...canSleep(state),
...canWork(state)
};
}
// Creating a user with composed functionalities
const user = createUser("Alice");
// Using the composed methods
user.eat("apple"); // Eating apple!
user.sleep(8); // Sleeping for 8 hours!
user.work(10); // Working for 10 hours!
// Checking the state after all actions
console.log(`${user.name}'s energy level: ${user.energy}`);