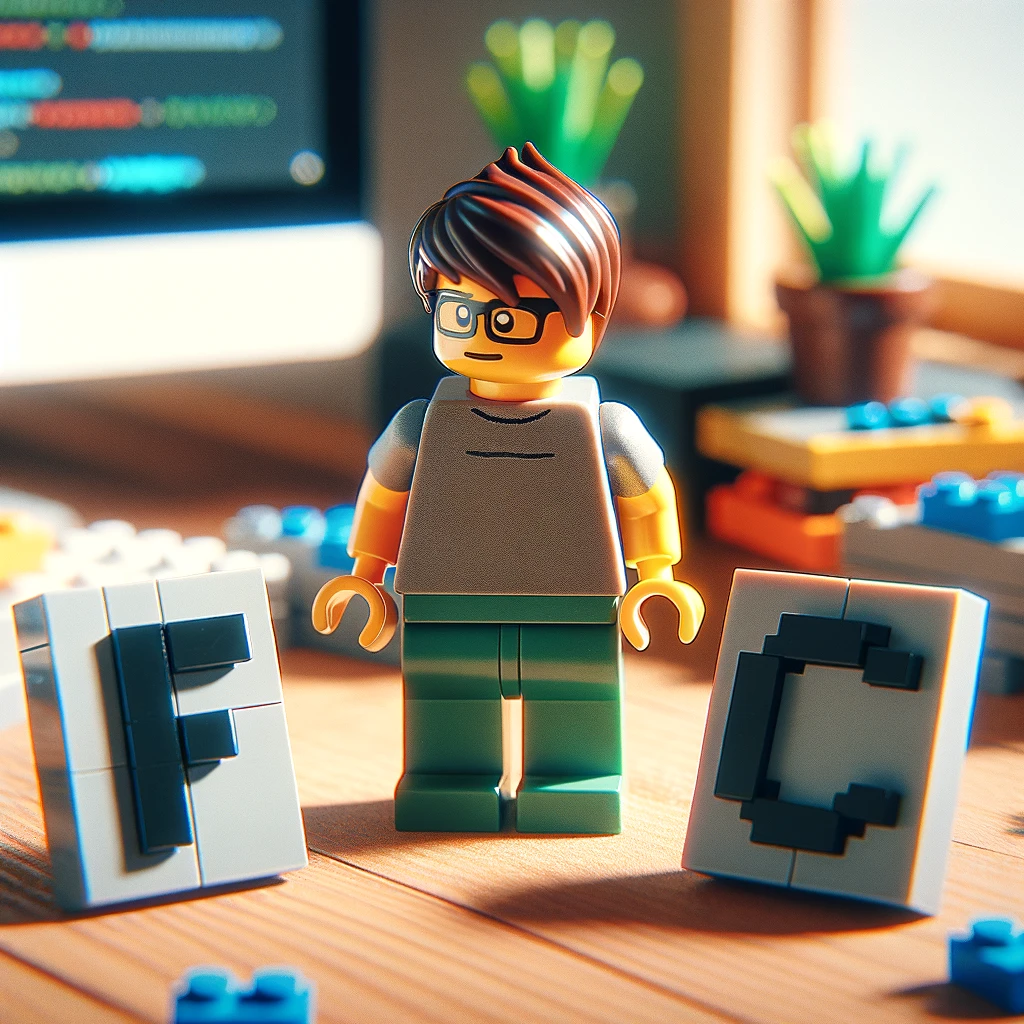
The usage of React.FC
or React.FunctionComponent
has been a subject of debate within the React community. Both approaches—using it or not—have their pros and cons.
Do not use React.FC
IMHO, do not use React.FC
or React.FunctionComponent
in your codebase, only if you know you are using children props.
Pros and cons
Pros
- Explicit Return Type: It makes it clear that the function is a React functional component.
- Children Prop:
React.FC
automatically includes thechildren
prop, so you don't have to define it yourself.
Cons
- Implicit Children: Automatically including
children
may be undesired if your component doesn't intend to handle children. - Generic Props: Using generics with
React.FC
can be less straightforward. - Possibly Redundant: If you're already typing your component props, the
React.FC
can be seen as redundant.
Alternative
Given these pros and cons, some projects and developers prefer to type their functional components without using React.FC
, like so:
without React.FC
interface Props {
message: string;
}
const MyComponent = ({ message }: Props) => {
return <div>{message}</div>;
};
This gives you more control over which props your component should accept, and it avoids the implicit inclusion of children
if it's not needed.
There isn't a single "standard approach" within the community; it's often a matter of team preference and the specific requirements of your project.