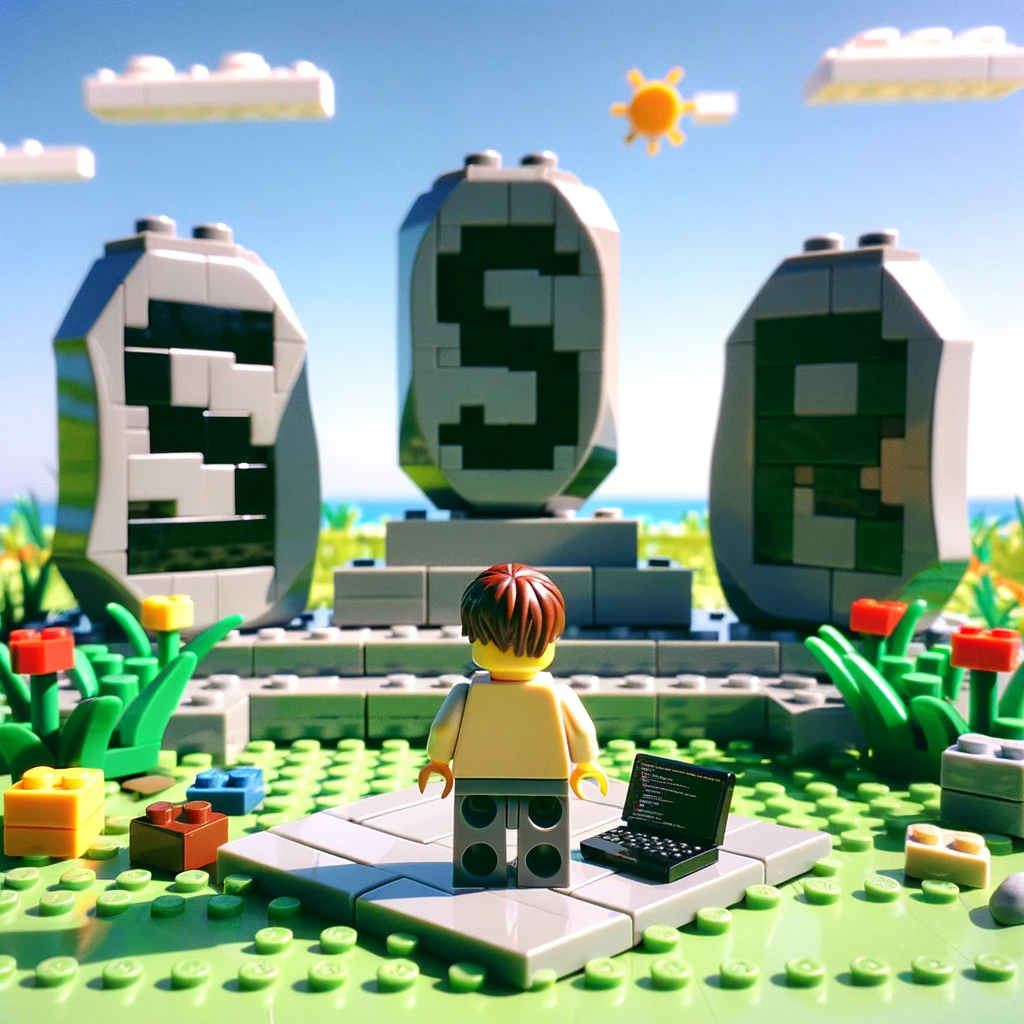
SSR stands for Server-Side Rendering. It's a technique used in web development where the content of a web page is generated on the server instead of in the user's browser.
SSR stands for Server-Side Rendering. It's a technique used in web development where the content of a web page is generated on the server instead of in the user's browser. This differs from client-side rendering (CSR), where JavaScript runs in the browser to produce the page's content dynamically.
Advantagesโ
In the context of React (a popular JavaScript library for building user interfaces), SSR means rendering components on the server into HTML, which is then sent to the browser. This process has several key advantages:
- Faster Initial Page Load: The browser receives a fully rendered page, so the content is visible and accessible immediately, even before all the JavaScript is downloaded and parsed. This can lead to better user experience, especially on slower connections.
- SEO Benefits: Search engines can more easily index the content because it's already rendered in the HTML. This is particularly beneficial for content-heavy sites where SEO is a priority.
- Improved Performance on Slow Devices: Because the server does the heavy lifting of rendering, devices with lower processing power don't have to work as hard, leading to a smoother experience.
Challengesโ
However, there are also challenges with SSR:
- Complexity: Implementing SSR can be more complex than client-side rendering, especially when dealing with data fetching, state management, and routing.
- Server Load: It can increase the load on your server, as it has to render pages for each request.
- Initial Load vs. Interactivity: While the initial content loads faster, the page can't become interactive until the JavaScript bundle is downloaded and executed.
Popular frameworks like Next.js simplify the implementation of SSR in React applications by providing a framework around React that supports SSR out of the box.
Is only the first request one done in SSR?โ
In a typical Server-Side Rendering (SSR) setup, yes, only the first request is rendered on the server.
How it generally worksโ
- First Request: When a user first visits a web page, the server generates the HTML for the page and sends it to the user's browser. This HTML includes the fully rendered page structure, along with any initial data loaded during the rendering process.
- Subsequent Navigation: After the initial page load, when the user navigates to other pages or interacts with the site, these actions are typically handled client-side. JavaScript (often bundled with frameworks like React) takes over and handles these interactions dynamically in the browser. This is known as Client-Side Rendering (CSR).
Hybrid Approachโ
Some applications use a hybrid approach where certain pages or components are server-rendered, while others are client-rendered. This is often used in scenarios where some parts of the application benefit significantly from SSR (like for SEO or performance), while others are more dynamic and suited for CSR.
Full-Page Refreshesโ
If there's a full-page refresh (like when the user hits the refresh button or enters a new URL), the server again renders the requested page and sends it to the browser.
This behavior leverages the strengths of both server-side and client-side rendering, offering a balance between initial load performance (benefiting from SSR) and dynamic, interactive user experiences (using CSR).
Tools like Next.js for React make managing this combination more straightforward by handling a lot of the complexity behind the scenes.
How is it done?โ
Implementing Server-Side Rendering (SSR) in a web application, particularly with React, involves several key steps. Let's break it down:
1. Server Setupโ
Node.js Server: You need a Node.js environment to run JavaScript on the server. This is because React, a JavaScript library, needs a JavaScript environment to execute.
Express.js: Often, an Express.js server is used to handle HTTP requests. Express is a flexible Node.js web application framework that provides a robust set of features for web applications.
2. Rendering React Components on the Serverโ
ReactDOMServer: React provides ReactDOMServer, which allows you to render components to static markup (typically HTML). You use methods like ReactDOMServer.renderToString() or ReactDOMServer.renderToStaticMarkup() to turn your React components into HTML strings.
Data Fetching: For data-driven applications, you might need to fetch data from APIs or databases. This is done before rendering the component so that the rendered HTML already includes the necessary data.
3. Sending HTML to the Clientโ
Response to Client: The server sends the rendered HTML as a response to the client's request. This response includes the full page structure, ready to be displayed in the browser.
Linking Client-Side JavaScript: The HTML should link to JavaScript bundles that enable React to take over in the browser for subsequent client-side interactions.
4. Hydrationโ
Hydration Process: Once the HTML is loaded and the JavaScript bundle is executed, React 'hydrates' the application. This means it attaches event listeners and enables the React components to become interactive. The term 'hydration' is used because the client-side React app essentially "fills up" the static content with interactivity.
Example with Next.jsโ
Frameworks like Next.js abstract much of this complexity. Here's a simplified view of how it works with Next.js:
- Page Creation: Create pages in the pages directory. Next.js handles the routing based on the file names.
- Data Fetching: Implement functions like getServerSideProps for server-side data fetching.
- Automatic Rendering: Next.js automatically renders these pages on the server on each request.
- Linking and Hydration: Next.js takes care of linking the necessary JavaScript and the hydration process.
Challenges and Considerationsโ
- State Management: Ensuring the server-rendered content and client-side state are in sync can be challenging.
- Performance Optimization: Server load and response time need to be optimized, especially for high-traffic applications.
- SEO and Accessibility: Server-rendered content improves SEO and can enhance accessibility, but it needs to be correctly implemented.
Implementing SSR, especially in complex applications, requires careful planning and consideration of how it will impact both the development process and the end-user experience.