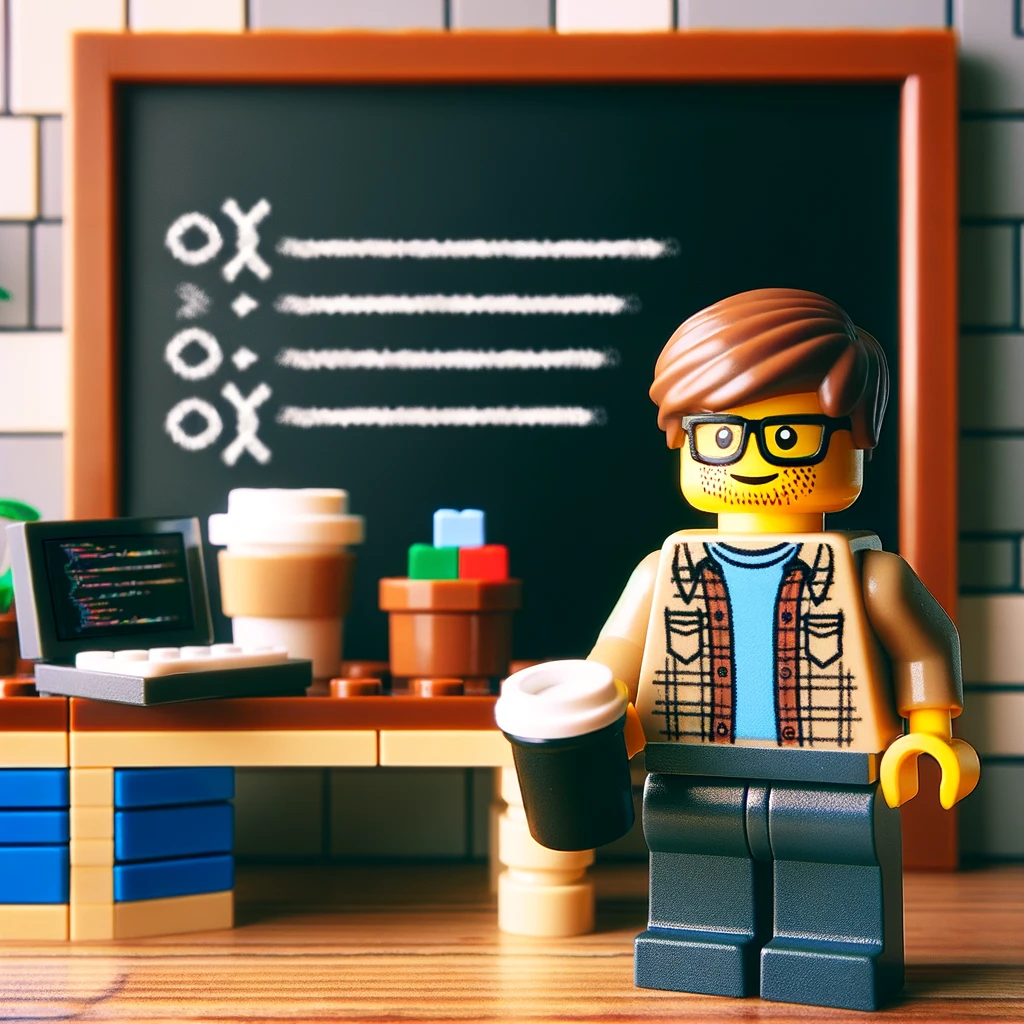
Recently, we had an in-depth discussion on structuring React applications using a layered architectural approach. This method aligns with fundamental software architecture principles such as the Single Responsibility Principle (SRP) and the Single Source of Truth (SSoT). It shares similarities with Clean Architecture and Layered Architecture and, interestingly, shows parallels with the Model-View-Controller (MVC) pattern in some respects. We are also applying Dependency Inversion principle (DIP), depending on abstractions rather than concrete implementations of lower-level modules.
- Benefits: The architecture offers clear separation of concerns, encapsulation, reusability, and scalability.
- Considerations: Potential complexity, performance implications of using Redux, and the learning curve associated with Redux and thunks.
Layered Architectural Strategy in Reactโ
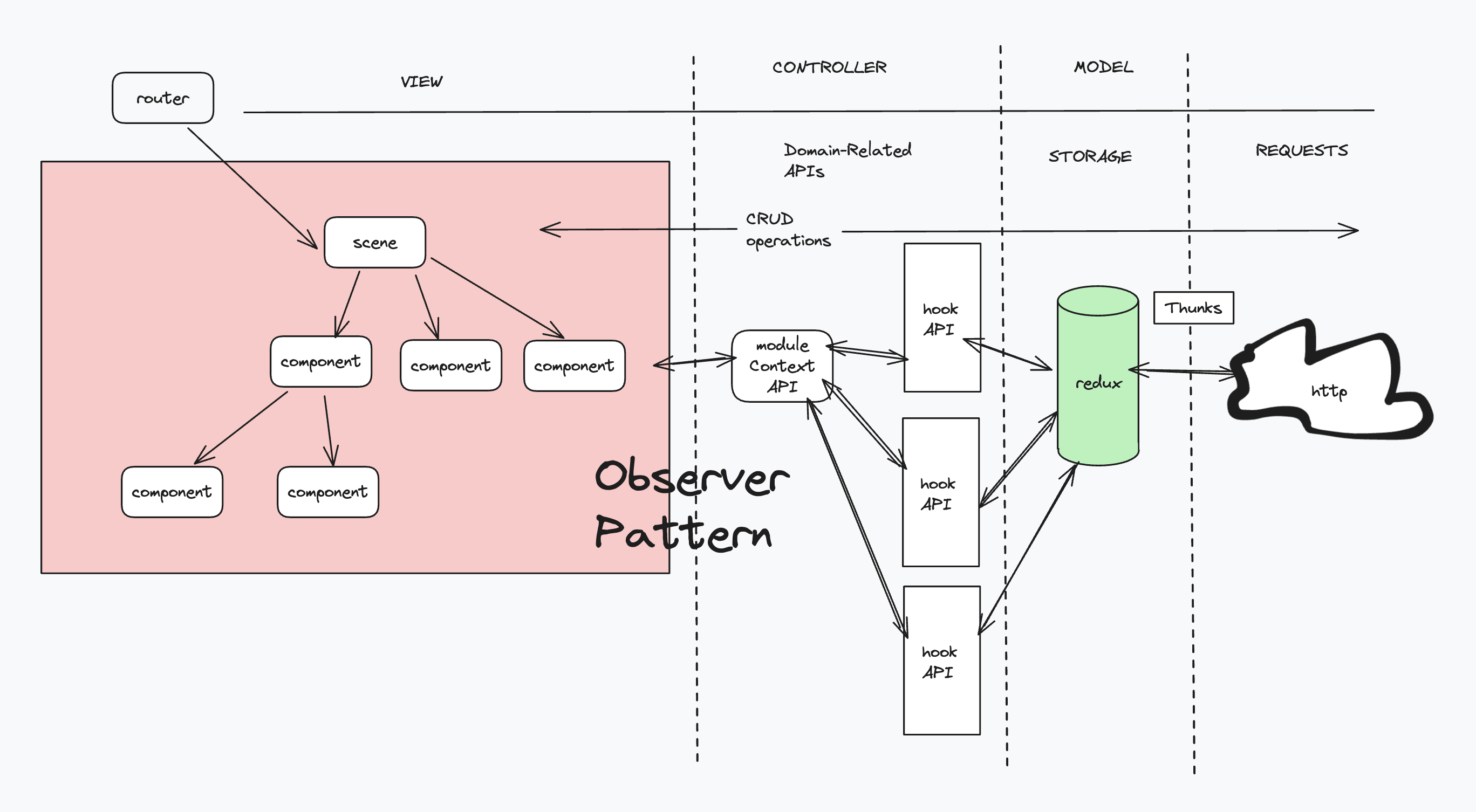
We can break down our approach into several distinct layers:
Low-Level Modules (Data Storage and HTTP Handling)
- HTTP Request Handling (Layer 1): This layer is dedicated to managing HTTP requests. It handles API interactions, error handling, and potentially data formatting.
- Data Storage (Layer 2 - Model): Acting as the Single Source of Truth, this layer abstracts the data storage and retrieval processes. It ensures consistent data modeling throughout the application.
High-Level Modules (Business Logic and React Components)
- Business Logic (Layer 3 - Controller): This layer is responsible for the application's business logic. It operates on the data provided by the second layer.
- React Components (Layer 4 - View): Analogous to the View in MVC, these components manage the presentation layer. They render the UI, present data, and respond to state changes, similar to how a View in MVC reflects data updates.
To achieve true dependency inversion, define interfaces in the higher-level modules (business logic). Lower-level modules (HTTP handling, data storage) should implement these interfaces.
This approach decouples the specifics of data retrieval and external interactions from the business logic, increasing modularity and testability.
React Custom Hooks as Abstractions (DIP)โ
Custom hooks should serve as the bridge between React components and the business logic. They encapsulate interactions with the Redux store and other business logic, adhering to the dependency inversion principle.
- Interface Design: When designing interfaces, focus on the needs of the business logic, not the details of lower-level implementations.
- Dependency Injection: Utilize dependency injection to provide concrete implementations of these interfaces to higher-level modules. This can be achieved using React's context system or custom hooks.
Example: Redux Storeโ
While the store manages application state, it should be interacted with through abstract interfaces or custom hooks, ensuring components are not directly dependent on the store's concrete implementation.
MVC Parallel and React/Redux Specificsโ
This structure somewhat resembles MVC. However, it's essential to recognize key differences in React/Redux architectures. React's functional approach and Redux's unidirectional data flow diverge from the bidirectional flow in traditional MVC. While this comparison helps in understanding, it doesn't fully encompass the uniqueness of React/Redux.
It's essential to recognize key differences in React/Redux architectures. React's functional approach and Redux's unidirectional data flow diverge from the bidirectional flow in traditional MVC. While this comparison helps in understanding, it doesn't fully encompass the uniqueness of React/Redux.
Iteration and Integration with the React Ecosystemโ
We also looked into integrating React hooks, Redux, and thunks within this architecture:
- React Components: Function as the UI elements that interact with business logic via custom hooks. They remain unaware of the underlying state management mechanism.
- Custom Hooks: Serve as an interface to the Redux store and business logic layer. These hooks simplify component logic, promote reusability, and are agnostic to the state management mechanism.
- Redux Store: Acts as the central state management system. It updates in response to actions or asynchronous operations triggered by thunks.
- Thunks: Handle side effects like API calls. Thunks execute HTTP requests, manage responses, and dispatch actions to update the Redux store.
- HTTP Layer: Manages external HTTP communications, including API calls and error handling.
Applying Dependency Inversionโ
- High-Level Modules: Focus on abstracted business logic.
- Low-Level Modules: Concern themselves with data storage, independent of high-level business logic.
Abstract Interface Between HTTP and Thunksโ
We've introduced an abstract interface to separate the HTTP layer from thunks, boosting flexibility and testability.
React Hooks and Store Subscriptionโ
Discussing React hooks and their role in our architecture:
- React Hooks and Rendering: Hooks like
useEffect
and custom hooks utilize React's rendering capabilities. They allow components to "subscribe" to changes in the Redux store. - Automatic Updates: When the store's state changes, React's rendering engine automatically updates the subscribed components. This ensures that the UI remains in sync with the current state.
- Efficiency and Performance: This mechanism of automatic subscription and updates is highly efficient. It minimizes the need for manual DOM updates and optimizes application performance.
Community Perspective and Relation to Established Patternsโ
- This strategy is in line with Clean Architecture and Layered Architecture principles, emphasizing separation of concerns and dependency management.
- It's popular in the web development community, especially for larger, more complex applications.
- The approach enhances maintainability, scalability, and testability - critical aspects of modern software development.
Conclusionโ
This layered architectural approach, integrated with the React ecosystem (hooks, Redux, thunks), establishes a robust, maintainable, and scalable framework for React applications. It conforms to modern development practices, striking a balance between maintainability and practical performance considerations. Like any architectural decision, it's important to weigh the complexity against the application's needs and the team's skill set.