SOLID Principles
SOLID is an acronym for five design principles intended to make software designs more understandable, flexible, and maintainable. It's particularly useful in object-oriented programming and helps with reducing dependencies, making systems easier to understand, modify, and extend. Here are the five principles:
-
Single Responsibility Principle (SRP):
- What it states: A class should have one, and only one, reason to change.
- Meaning: Each class should be responsible for a single part of the functionality provided by the software, and that responsibility should be entirely encapsulated by the class.
-
Open/Closed Principle (OCP):
- What it states: Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
- Meaning: You should be able to add new functionality without changing the existing code. This is often achieved using interfaces or abstract classes.
-
Liskov Substitution Principle (LSP):
- What it states: Objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
- Meaning: Subclasses should override the parent classes in ways that do not break functionality from a client's point of view.
-
Interface Segregation Principle (ISP):
- What it states: No client should be forced to depend on methods it does not use.
- Meaning: Instead of one big interface, many client-specific interfaces are better. This principle leads to a system with many small, client-specific interfaces.
-
Dependency Inversion Principle (DIP):
- What it states: High-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
- Meaning: This principle is aimed at reducing the coupling between the software modules. Rather than high-level modules depending on low-level modules, both should depend on abstractions.
How SOLID Applies to Software Development:​
- Maintainability: Changes in software can be made more easily due to reduced dependencies.
- Flexibility: It's easier to add new features because the system is more modular.
- Understandability: With clear separation and modular design, code is easier to understand.
- Testability: Independent modules are easier to test in isolation.
Tips for Implementing SOLID Principles:​
- Start with a clear understanding of the problem and then carefully consider which principles apply to your specific situation.
- Refactor existing code incrementally, applying SOLID principles to areas that change frequently or seem particularly fragile.
- Use design patterns, many of which naturally lead to SOLID code, as they embody these principles.
History​
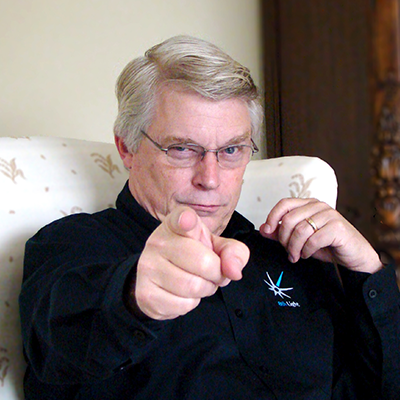
The principles are a subset of many principles promoted by American software engineer and instructor Robert C. Martin, first introduced in his 2000 paper Design Principles and Design Patterns discussing software rot.
SOLID applied to Functional Programming​
As software development trends increasingly embrace functional programming (FP), it's important to understand how SOLID principles, originally designed for object-oriented programming (OOP), fit into this paradigm shift. Here's a look at the relevance and application of each SOLID principle in the context of FP:
-
Single Responsibility Principle (SRP): In FP, this principle translates to having functions that do one thing and do it well. Functions should be small, focused, and reusable. This aligns well with the FP ethos of pure functions and avoiding side-effects.
-
Open/Closed Principle (OCP): FP naturally supports this principle through higher-order functions and function composition. Functions can be created in a way that allows new behavior to be introduced without changing existing functions. Additionally, FP's emphasis on immutability aligns with the idea of being closed for modification.
-
Liskov Substitution Principle (LSP): LSP is more relevant to OOP due to its focus on inheritance. FP tends to avoid inheritance in favor of composition. However, the core idea of LSP, which is about ensuring that functions or modules can be replaced with their subtypes or variations without affecting program correctness, still holds. In FP, this can be seen in the consistent use of function signatures and return types.
-
Interface Segregation Principle (ISP): FP naturally adheres to ISP since functions are generally small and specific. There's less emphasis on implementing interfaces as seen in OOP. Instead, FP focuses on creating small, reusable, and composable functions that clients can choose to use as needed.
-
Dependency Inversion Principle (DIP): In FP, DIP can be applied through the use of higher-order functions and dependency injection. Functions are passed as arguments, and dependencies can be injected, allowing for more flexible and testable code. This aligns with the idea of depending on abstractions rather than concrete implementations.
Conclusion​
While SOLID principles originated in the OOP domain, many of their core ideas are applicable and beneficial in functional programming, albeit in a different form. The principles of clear responsibilities, extension without modification, robustness to changes in specific implementations, and dependency on abstractions rather than concrete details are universal in software design, regardless of the programming paradigm.
As programming trends evolve, the underlying principles of good software design remain relevant, often manifesting in new patterns and practices suited to the paradigm in use.